jsp模糊查询分页代码最简单实现
时间: 2023-05-04 15:02:45 浏览: 313
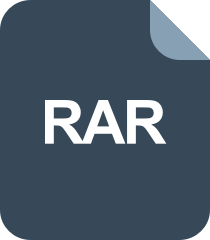
jsp带模糊查询的分页代码

对于JSP模糊查询分页的实现,可以采用以下简单的代码实现:
首先,在jsp页面中,定义相应的查询表单,包括输入查询关键字和选择页码等必要的组件。
接着,在后台的servlet中,获取相应的查询关键字,并结合查询语句实现模糊查询,同时根据选择的页码计算出需要查询的数据起始位置和结束位置,从而实现数据分页。
最后,将查询结果及分页信息传送到jsp页面中,结合相应的页面模板实现数据展示和分页导航。
具体的代码实现可参考以下示例:
1. JSP页面:
<form method="post" action="query.jsp">
<input type="text" name="keyword" placeholder="请输入查询关键字" required>
<button type="submit">查询</button>
</form>
<%-- 数据展示 --%>
<table>
<thead>
<tr>
<th>序号</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<% for(int i=0; i<list.size(); i++){ %>
<tr>
<td><%=i+1 %></td>
<td><%=list.get(i).getName() %></td>
<td><%=list.get(i).getAge() %></td>
<td><%=list.get(i).getGender() %></td>
</tr>
<% } %>
</tbody>
</table>
<%-- 分页导航 --%>
<% if(totalPage>0){ %>
<div class="pagination">
<% if(curPage>1){ %>
<a href="?keyword=<%=keyword %>&page=<%=curPage-1 %>" class="prev">«</a>
<% } %>
<% for(int i=1; i<=totalPage; i++){ %>
<% if(i==curPage){ %>
<span class="current"><%=i %></span>
<% }else{ %>
<a href="?keyword=<%=keyword %>&page=<%=i %>" class="page"><%=i %></a>
<% } %>
<% } %>
<% if(curPage<totalPage){ %>
<a href="?keyword=<%=keyword %>&page=<%=curPage+1 %>" class="next">»</a>
<% } %>
</div>
<% } %>
2. Servlet代码:
String keyword = request.getParameter("keyword");
int curPage = request.getParameter("page")==null ? 1 : Integer.valueOf(request.getParameter("page"));
int pageSize = 10;
int startIndex = (curPage-1)*pageSize;
int endIndex = curPage*pageSize-1;
List<Data> list = dao.query(keyword, startIndex, endIndex);
int totalCount = dao.count(keyword);
int totalPage = (totalCount-1)/pageSize+1;
request.setAttribute("list", list);
request.setAttribute("keyword", keyword);
request.setAttribute("curPage", curPage);
request.setAttribute("totalPage", totalPage);
request.getRequestDispatcher("query.jsp").forward(request, response);
3.DAO代码:
public List<Data> query(String keyword, int startIndex, int endIndex){
String sql = "select * from data where name like ? limit ?,?";
Object[] params = new Object[]{ '%'+keyword+'%', startIndex, endIndex };
return template.query(sql, new BeanPropertyRowMapper<Data>(Data.class), params);
}
public int count(String keyword){
String sql = "select count(*) from data where name like ?";
Object[] params = new Object[]{ '%'+keyword+'%' };
return template.queryForObject(sql, Integer.class, params);
}
需要注意的是,该示例代码使用了JavaWeb的常用组件,如servlet、JDBC和JSP等,同时也使用了一些常用的第三方jar包,如spring-jdbc和mysql-connector-java等,因此在使用前需确保相关环境和依赖都已配置完毕。同时,由于该示例只是展示了一种可能的实现方式,如果需要满足更多的实际需求,还需根据具体情况进行相应的调整和扩展。
阅读全文
相关推荐
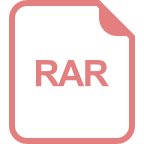
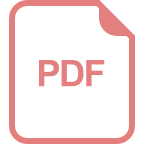
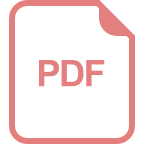
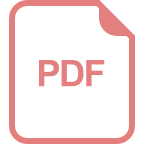
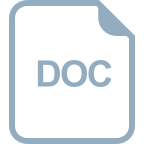
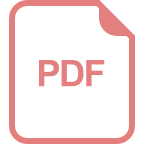
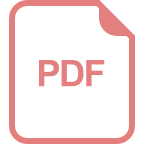
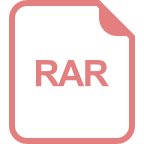
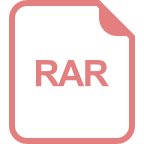
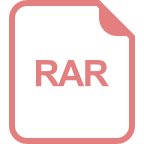
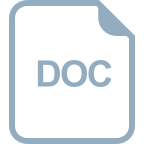



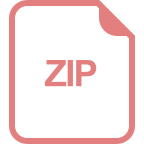
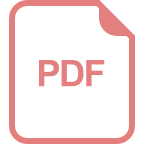