mp3d_find_frame用法
时间: 2023-10-10 10:11:16 浏览: 36
`mp3d_find_frame` 是一个函数,用于在 MP3 文件中查找帧同步字。下面是该函数的使用方法:
```c
int mp3d_find_frame(unsigned char *buf, int buf_size)
```
参数说明:
- `buf`:指向包含 MP3 数据的缓冲区的指针。
- `buf_size`:缓冲区的大小,以字节为单位。
返回值:
- 如果找到帧同步字,则返回帧的长度(以字节为单位)。
- 如果没有找到帧同步字,则返回 0。
使用示例:
```c
unsigned char *mp3_data; // 假设指向 MP3 数据的指针
int mp3_data_size; // 假设 MP3 数据的大小(以字节为单位)
int frame_size = mp3d_find_frame(mp3_data, mp3_data_size);
if (frame_size > 0) {
// 找到了帧同步字,可以处理这一帧的数据
// 比如可以使用 libmpg123 解码这一帧
} else {
// 没有找到帧同步字,可能需要等待更多数据
}
```
需要注意的是,该函数只能找到完整的 MP3 帧,如果缓冲区中的数据不足一帧,则函数会返回 0。因此,在处理 MP3 数据时,可能需要使用一个缓冲区,不断从文件中读取数据,直到找到一个完整的帧为止。
相关问题
avformat_find_stream_info使用方法
avformat_find_stream_info 是 FFmpeg 中用于获取媒体文件音视频流信息的函数,它的原型如下:
```
int avformat_find_stream_info(AVFormatContext *ic, AVDictionary **options);
```
其中,ic 是一个 AVFormatContext 结构体指针,表示要进行音视频流信息获取的媒体文件的格式上下文;options 是一个 AVDictionary 结构体指针,表示附加的选项参数,可选。
avformat_find_stream_info 函数的作用是获取媒体文件中的音视频流信息,并将信息存储在 AVFormatContext 结构体中。在调用此函数之后,可以通过遍历 AVFormatContext 结构体中的 streams 数组来获取每个音视频流的详细信息,包括编解码器参数、流的类型(音频或视频)、流的时长、帧率等。
使用 avformat_find_stream_info 函数时,需要先调用 avformat_open_input 函数打开要读取的媒体文件,并使用 avformat_alloc_context 函数创建一个 AVFormatContext 结构体。然后,可以使用 avformat_find_stream_info 函数获取音视频流信息。函数返回值表示是否获取成功,如果返回值小于 0,表示获取失败,可以通过 av_strerror 函数将错误码转换为错误信息进行查看。
下面是一个示例代码,演示了如何使用 avformat_find_stream_info 函数获取音视频流信息:
```c
AVFormatContext *fmt_ctx = NULL;
int ret = avformat_open_input(&fmt_ctx, "test.mp4", NULL, NULL);
if (ret < 0) {
// 打开媒体文件失败
return;
}
ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
// 获取音视频流信息失败
avformat_close_input(&fmt_ctx);
return;
}
// 遍历音视频流信息
for (int i = 0; i < fmt_ctx->nb_streams; i++) {
AVCodecParameters *codecpar = fmt_ctx->streams[i]->codecpar;
if (codecpar->codec_type == AVMEDIA_TYPE_AUDIO) {
// 音频流信息
printf("Audio Stream: sample_rate=%d, channels=%d\n", codecpar->sample_rate, codecpar->channels);
} else if (codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
// 视频流信息
printf("Video Stream: width=%d, height=%d, fps=%f\n", codecpar->width, codecpar->height, av_q2d(fmt_ctx->streams[i]->avg_frame_rate));
}
}
avformat_close_input(&fmt_ctx);
```
openmv中find_color_blob的用法
`find_color_blob()` 是 OpenMV 中的一个图像处理函数,用于在图像中查找指定颜色的物体。该函数的语法如下:
```python
find_color_blob(color, threshold=(50, 50, 50), roi=None, merge=True)
```
参数说明:
- `color`:要查找的颜色,可以是 RGB 三元组或者 HSV 三元组。
- `threshold`:颜色阈值,用于指定颜色的容差范围。默认为 `(50, 50, 50)`。
- `roi`:感兴趣区域(ROI),用于指定查找的图像区域。默认为整个图像。
- `merge`:是否将找到的颜色块进行合并。默认为 `True`。
该函数返回一个 `blob` 对象,代表找到的颜色块。可以通过该对象的各种属性和方法获取颜色块的位置、大小、颜色等信息。以下是一个简单的示例代码:
```python
import sensor, image
# 初始化摄像头和图像传感器
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
# 主循环
while True:
# 拍摄一张图片
img = sensor.snapshot()
# 查找红色物体
blob = img.find_color_blob((255, 0, 0))
# 如果找到了红色物体
if blob:
# 在串口上打印物体的位置和大小
print("x = %d, y = %d, w = %d, h = %d" % (blob.x(), blob.y(), blob.w(), blob.h()))
# 在屏幕上显示图像
img.draw_rectangle(0, 0, 80, 60, color = (255, 255, 255))
img.draw_string(5, 5, "Hello, OpenMV!", color = (0, 0, 255))
```
这个代码运行后,会实时监测摄像头画面中是否有红色物体,如果有,就会在串口上打印出物体的位置和大小。同时,还会在屏幕上显示一个矩形框和一行文字。
相关推荐
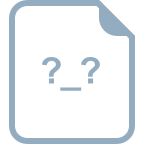












