使用feign调用接口获取文件流,接收的时候变为了LinkedHashMap类型
时间: 2024-03-15 14:44:13 浏览: 18
Feign是一个HTTP客户端框架,它可以将接口定义转换成HTTP请求。在使用Feign调用接口获取文件流时,需要使用`ResponseEntity<Resource>`类型作为返回值类型,其中Resource是Spring中封装了文件流的类。
如果你接收到的是LinkedHashMap类型,可能是因为Feign默认使用Jackson将HTTP响应转换为JSON对象。在这种情况下,你需要在Feign接口的方法上添加`@ResponseContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE)`注解,告诉Feign将响应的内容解析为字节流。例如:
```
@RequestLine("GET /download")
@Headers("Accept: application/octet-stream")
@ResponseContentType(MediaType.APPLICATION_OCTET_STREAM_VALUE)
ResponseEntity<Resource> download();
```
这样,你就可以成功地将文件流作为ResponseEntity<Resource>类型接收。
相关问题
java如何使用feign调用接口
Feign是一个声明式、模板化的HTTP客户端,用于简化HTTP API客户端的开发。使用Feign可以方便地调用其他服务的REST接口,下面是Java中使用Feign调用接口的步骤:
1. 添加Feign依赖
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
```
2. 创建Feign客户端接口
```java
@FeignClient(name = "service-name")
public interface ServiceClient {
@GetMapping("/api/{id}")
Result getById(@PathVariable("id") Long id);
}
```
其中,@FeignClient注解用于声明一个Feign客户端,name属性指定了服务名称。getById方法对应了服务接口中的一个API,@GetMapping注解指定了API的请求方式和路径。
3. 注入Feign客户端
```java
@Autowired
private ServiceClient serviceClient;
```
4. 调用Feign客户端
```java
Result result = serviceClient.getById(id);
```
其中,id为API的参数。
以上就是Java中使用Feign调用接口的基本步骤。需要注意的是,Feign默认使用的是Spring的RestTemplate发送HTTP请求,也可以使用其他HTTP客户端替换RestTemplate。
feign远程调用传文件流
Feign是一个声明式的Web服务客户端,它使得编写Web服务客户端变得更加容易。在Feign中,我们只需要定义一个接口并注解,即可完成对Web服务接口的绑定。在进行远程调用时,我们可以使用Feign的@RequestPart注解来传递文件流。具体步骤如下:
1.在Feign接口中定义远程调用方法,使用@RequestPart注解来传递文件流,例如:
```java
@RequestLine("POST /upload")
@Headers("Content-Type: multipart/form-data")
void uploadFile(@RequestPart("file") MultipartFile file);
```
2.在Feign客户端中开启对multipart/form-data的支持,例如:
```java
@Configuration
public class FeignMultipartSupportConfig {
@Bean
public Encoder feignFormEncoder() {
return new SpringFormEncoder();
}
}
```
3.在调用Feign接口时,将文件流作为参数传递给远程方法,例如:
```java
@Autowired
private FileFeignClient fileFeignClient;
public void uploadFile(MultipartFile file) {
fileFeignClient.uploadFile(file);
}
```
相关推荐
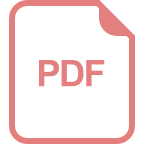
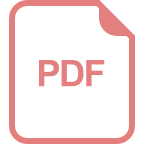












