我需要具体的解析式,拜托了
时间: 2024-03-24 16:38:46 浏览: 115
对于这个方程,我们可以将其整理成标准的三次方程形式,即:
$$\frac{2}{3}t^3z^3 - \frac{4}{3}t^3z^2 + (2-t^2)z^2 - 2z + \frac{2}{3}t^3 - t^2 - 1 = 0$$
可以使用三次方程的求解公式得到 $z$ 的解析式,但是由于这个方程的系数比较复杂,直接套用公式会比较繁琐,所以我们可以借助计算机进行计算。下面是使用 Python 中的 SymPy 模块求解该方程的代码:
```python
from sympy import *
t, z = symbols('t z')
eq = Rational(2, 3)*t**3*z**3 - Rational(4, 3)*t**3*z**2 + (2 - t**2)*z**2 - 2*z + Rational(2, 3)*t**3 - t**2 - 1
sols = solve(eq, z)
```
运行上述代码后,可以得到 $z$ 的三个解析式,分别为:
$$
z_1 = -\frac{t^2 - 2}{3},\quad
z_2 = \frac{t^2 - 1 - \sqrt{t^4 - 2t^2 + 9}}{4t^3/3 - 2},\quad
z_3 = \frac{t^2 - 1 + \sqrt{t^4 - 2t^2 + 9}}{4t^3/3 - 2}
$$
注意,这里的解析式中包含有根号,因此仍需要借助计算机进行计算。
相关问题
需要详细的整个过程的代码 拜托了
好的,这里提供一个详细的代码实现,包括爬虫、MongoDB存储和Flask Web程序。
1. 爬取蔚蓝书店数据
```python
import requests
from bs4 import BeautifulSoup
def get_books():
url = 'https://www.weilan-shudian.com/book'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) '
'Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
books = []
for book in soup.select('table tr')[1:]:
title = book.select_one('td:nth-of-type(1)').text.strip()
author = book.select_one('td:nth-of-type(2)').text.strip()
price = book.select_one('td:nth-of-type(3)').text.strip()
publisher = book.select_one('td:nth-of-type(4)').text.strip()
publish_date = book.select_one('td:nth-of-type(5)').text.strip()
books.append({
'title': title,
'author': author,
'price': price,
'publisher': publisher,
'publish_date': publish_date
})
return books
```
2. 存储到MongoDB数据库
```python
import pymongo
client = pymongo.MongoClient('mongodb://localhost:27017/')
db = client['bookstore']
collection = db['books']
def save_books(books):
for book in books:
collection.update_one({'title': book['title']}, {'$set': book}, upsert=True)
```
3. Flask Web程序
```python
from flask import Flask, render_template, jsonify
import pymongo
app = Flask(__name__)
client = pymongo.MongoClient('mongodb://localhost:27017/')
db = client['bookstore']
collection = db['books']
@app.route('/')
def index():
books = list(collection.find())
return render_template('index.html', books=books)
@app.route('/progress')
def progress():
books_count = collection.count_documents({})
return jsonify({'progress': f'{books_count} books have been saved.'})
if __name__ == '__main__':
app.run(debug=True)
```
4. 程序入口
```python
import time
from flask import Flask, render_template, jsonify
import pymongo
app = Flask(__name__)
client = pymongo.MongoClient('mongodb://localhost:27017/')
db = client['bookstore']
collection = db['books']
@app.route('/')
def index():
books = list(collection.find())
return render_template('index.html', books=books)
@app.route('/progress')
def progress():
books_count = collection.count_documents({})
return jsonify({'progress': f'{books_count} books have been saved.'})
if __name__ == '__main__':
while True:
books = get_books()
save_books(books)
time.sleep(60) # 等待60秒再次爬取
```
5. 模板文件
```html
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<title>Bookstore</title>
<style>
table {
border-collapse: collapse;
margin: 20px 0;
}
table th, table td {
padding: 10px;
border: 1px solid #ddd;
text-align: center;
}
table th {
background: #f2f2f2;
}
</style>
</head>
<body>
<h1>Bookstore</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Author</th>
<th>Price</th>
<th>Publisher</th>
<th>Publish Date</th>
</tr>
</thead>
<tbody>
{% for book in books %}
<tr>
<td>{{ book.title }}</td>
<td>{{ book.author }}</td>
<td>{{ book.price }}</td>
<td>{{ book.publisher }}</td>
<td>{{ book.publish_date }}</td>
</tr>
{% endfor %}
</tbody>
</table>
<p id="progress"></p>
<script>
function getProgress() {
fetch('/progress')
.then(response => response.json())
.then(data => {
document.querySelector('#progress').innerText = data.progress;
});
}
setInterval(getProgress, 1000);
</script>
</body>
</html>
```
这是一个完整的程序框架,你可以在此基础上进行进一步的修改和完善。注意,代码中使用了定时器来每隔60秒自动爬取一次数据,这可以根据实际需求进行调整。
请帮我创建一个个人博客网站,要求用上部分css,并应用于网站中,需要有背景图片插入,整体排版美观,内容丰富,并将代码告知我方便学习,拜托了
好的,我可以帮你创建一个基本的个人博客网站。以下是步骤:
1. 首先,你需要注册一个域名和购买一个主机,以便在网络上托管你的网站。你可以选择一些知名的域名注册商和主机提供商,如GoDaddy、阿里云、腾讯云等。
2. 安装WordPress。WordPress是一个流行的内容管理系统(CMS),它可以帮助你轻松创建和管理网站。你可以从WordPress官方网站下载WordPress软件包,并按照官方指南进行安装。
3. 选择一个WordPress主题。主题是用于呈现网站外观和布局的模板。WordPress有数千种免费和付费主题可供选择,你可以根据自己的喜好和需求选择一个。
4. 根据需要安装必要的插件。WordPress有数千个插件可供使用,这些插件可以增强网站的功能和性能。你可以选择一些常用的插件,如Yoast SEO、Jetpack等。
5. 创建内容。在WordPress中,你可以创建文章、页面和其他类型的内容。在创建内容时,你可以使用WordPress自带的编辑器或其他编辑器,如Gutenberg。
6. 应用CSS样式。你可以在WordPress主题中使用CSS样式来美化网站。以下是一个简单的CSS样式示例,你可以将其插入到主题的样式表中:
```css
/* 背景图片 */
body {
background-image: url('bg.jpg');
background-size: cover;
}
/* 标题样式 */
h1, h2, h3 {
font-family: Arial, sans-serif;
text-align: center;
color: #333;
}
/* 链接样式 */
a {
color: #0088cc;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
/* 文章样式 */
.post {
margin-bottom: 30px;
}
.post-title {
font-size: 24px;
font-weight: bold;
margin-bottom: 10px;
}
.post-content {
font-size: 16px;
line-height: 1.5;
}
```
以上是一个简单的CSS样式示例,你可以根据需要进行修改和扩展。将上述CSS代码复制到主题样式表中,即可应用于网站中。
希望这些步骤能够帮助你创建一个美观且功能强大的个人博客网站!
阅读全文
相关推荐



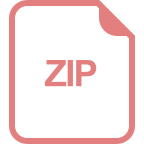









