js byte[] 和string 相互转换
时间: 2023-12-26 18:05:48 浏览: 31
黑盒测试和白盒测试是软件测试中常用的两种方法,它们的区别在于测试人员的测试方法和测试目的不同。
1. 黑盒测试
黑盒测试是一种测试方法,测试人员只关注被测试软件的输入和输出,而不关注软件的内部结构和代码。测试人员并不知道软件的内部工作原理,只是根据需求规格说明书和测试计划对软件进行测试,以验证软件是否符合需求规格说明书中的要求。
黑盒测试的优点是可以更好地模拟用户的使用环境,从而发现更多的问题。缺点是无法深入了解软件的内部结构和代码,并且测试结果可能无法精确地定位问题。
2. 白盒测试
白盒测试是一种测试方法,测试人员需要对被测试软件的内部结构和代码有一定的了解,以便更好地测试软件的各个部分。测试人员可以通过对软件的代码进行分析和测试,以验证软件是否符合设计和编码规范,并且能够发现潜在的问题和漏洞。
白盒测试的优点是可以深入了解软件的内部结构和代码,从而发现更多的问题,并且测试结果可以精确地定位问题。缺点是需要专业的测试人员和测试工具,成本较高。
总体来说,黑盒测试和白盒测试都是有效的测试方法,测试人员可以根据具体的测试需求和测试目的选择合适的测试方法。
相关问题
js byte[]和string转化
在JavaScript中,可以通过以下方式将byte[]转换为string:
1. 使用TextDecoder()构造函数将byte[]转换为string:
```javascript
const decoder = new TextDecoder('utf-8');
const byteArray = new Uint8Array([72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]);
const str = decoder.decode(byteArray);
console.log(str); // Output: Hello World
```
2. 使用String.fromCharCode()方法将byte[]转换为string:
```javascript
const byteArray = new Uint8Array([72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]);
const str = String.fromCharCode.apply(null, byteArray);
console.log(str); // Output: Hello World
```
将string转换为byte[]可以通过以下方式实现:
1. 使用TextEncoder()构造函数将string转换为byte[]:
```javascript
const encoder = new TextEncoder();
const str = 'Hello World';
const byteArray = encoder.encode(str);
console.log(byteArray); // Output: Uint8Array(11) [72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100]
```
2. 使用ArrayBuffer()构造函数将string转换为byte[]:
```javascript
const str = 'Hello World';
const buffer = new ArrayBuffer(str.length * 2); // 2 bytes per character
const byteArray = new Uint8Array(buffer);
for (let i = 0; i < str.length; i++) {
byteArray[i] = str.charCodeAt(i);
}
console.log(byteArray); // Output: Uint8Array(22) [72, 0, 101, 0, 108, 0, 108, 0, 111, 0, 32, 0, 87, 0, 111, 0, 114, 0, 108, 0, 100, 0]
```
byte数组转string js
在 JavaScript 中将 byte 数组转换为字符串可以使用以下代码:
```javascript
function byteArrayToString(byteArray) {
var result = '';
for (var i = 0; i < byteArray.length; i++) {
result += String.fromCharCode(byteArray[i]);
}
return result;
}
// 示例
var byteArray = new Uint8Array([72, 101, 108, 108, 111]); // Hello 的 UTF-8 编码
var str = byteArrayToString(byteArray); // 转换为字符串
console.log(str); // 输出 Hello
```
这里使用了 `Uint8Array` 类型来存储 byte 数组,`String.fromCharCode` 方法将每个字节转换为对应的字符,并拼接到结果字符串中。最后返回结果字符串即可。
相关推荐
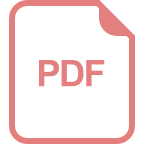
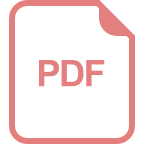
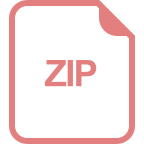












