c语言 宏定义 字符串转大写r
时间: 2024-01-02 16:05:19 浏览: 178
可以使用宏定义来将字符串转换为大写,如下所示:
```c
#define TO_UPPER(str) do { \
char *p = str; \
while (*p) { \
if (*p >= 'a' && *p <= 'z') { \
*p -= 32; \
} \
p++; \
} \
} while (0)
```
使用方式如下:
```c
char str[] = "hello world";
TO_UPPER(str);
printf("%s\n", str); // 输出 HELLO WORLD
```
这里使用了一个 do-while(0) 的技巧,可以避免宏定义在展开时产生意外的副作用。
相关问题
java宏定义字符串
在Java中,没有像C语言那样的宏定义。但是你可以使用常量来代替宏定义字符串。常量可以使用`final`关键字进行定义,以确保它们的值在定义后不可更改。
下面是一个示例代码:
```java
public class Constants {
public static final String MY_STRING = "Hello, world!";
}
```
你可以在其他类中使用`Constants.MY_STRING`来引用这个字符串常量。例如:
```java
public class Main {
public static void main(String[] args) {
System.out.println(Constants.MY_STRING);
}
}
```
这将打印出`Hello, world!`。请注意,这里的常量使用了大写命名规范,这是Java编码约定的一种常见做法。
如何用C语言编程来统计字符串中大写字母、数字和空格的数量?
在C语言中,你可以使用标准库函数来实现这个功能。首先,你需要定义三个变量分别用于存储大写字母、数字和空格的数量。然后遍历字符串,对每个字符进行判断并更新计数。
以下是一个简单的示例:
```c
#include <stdio.h>
#include <ctype.h> // 包含ctype.h头文件,它包含了处理字符类别相关的宏
int countUpperCase(char *str) {
int uppercase_count = 0;
for(int i = 0; str[i] != '\0'; i++) { // 遍历直到遇到字符串结束符'\0'
if(isupper(str[i])) { // 使用isupper检查是否为大写字母
uppercase_count++;
}
}
return uppercase_count;
}
int countNumbers(char *str) {
int number_count = 0;
for(int i = 0; str[i] != '\0'; i++) {
if(isdigit(str[i])) { // 使用isdigit检查是否为数字
number_count++;
}
}
return number_count;
}
int countSpaces(char *str) {
int space_count = 0;
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] == ' ') { // 直接比较是否为空格
space_count++;
}
}
return space_count;
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // 从用户输入获取字符串
// 移除fgets留下的换行符
size_t len = strlen(input);
if (input[len - 1] == '\n') {
input[len - 1] = '\0';
}
int upper = countUpperCase(input);
int num = countNumbers(input);
int space = countSpaces(input);
printf("Number of uppercase letters: %d\n", upper);
printf("Number of numbers: %d\n", num);
printf("Number of spaces: %d\n", space);
return 0;
}
```
阅读全文
相关推荐
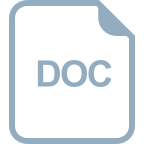












