用javafx写一个迷宫游戏
时间: 2023-07-01 09:19:35 浏览: 148
好的,我可以为您提供一些关于使用JavaFX编写迷宫游戏的指导。
首先,您需要创建一个JavaFX项目并导入所需的库。然后,您可以创建一个迷宫游戏的场景。场景可以包括一个迷宫地图、玩家角色、怪物、奖励和其他元素。
接下来,您需要编写代码来控制玩家的移动和与其他元素的交互。您可以通过键盘事件来控制角色的移动,例如按下方向键来移动角色。您还可以编写代码来检测角色是否与怪物或奖励接触,并根据游戏规则进行处理。
在编写代码时,您可以使用JavaFX提供的各种UI组件来创建游戏界面,例如按钮、文本框和标签。您还可以使用JavaFX提供的动画和过渡效果来增强游戏的视觉效果。
最后,您可以通过编写代码来保存和加载游戏状态,以便玩家可以在下次游戏时继续他们的进度。您可以使用Java的持久化技术,例如序列化或数据库,来实现此功能。
希望这些指导可以帮助您编写出一个很棒的迷宫游戏。如果您需要更多帮助,请随时问我。
相关问题
用javafx写一个迷宫游戏要求有自动寻路功能
好的,以下是一个简单的迷宫游戏实现方案,其中包括自动寻路功能。需要注意的是,这只是一个初步的示例程序,实际开发中可能需要进行更多的优化和改进。
首先,我们需要设计迷宫地图的数据结构。我们可以用一个二维数组来表示迷宫,其中0表示通路,1表示墙壁。例如,下面的数组表示一个5x5的迷宫地图:
```
int[][] maze = {
{1, 1, 1, 1, 1},
{1, 0, 0, 0, 1},
{1, 0, 1, 0, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
};
```
然后,我们可以用JavaFX来实现迷宫游戏的界面。我们可以使用一个GridPane来展示迷宫地图。
```
GridPane gridPane = new GridPane();
for (int i = 0; i < maze.length; i++) {
for (int j = 0; j < maze[0].length; j++) {
Rectangle rect = new Rectangle(50, 50);
if (maze[i][j] == 1) {
rect.setFill(Color.BLACK);
} else {
rect.setFill(Color.WHITE);
}
gridPane.add(rect, j, i);
}
}
```
接着,我们需要实现自动寻路算法。这里我们可以使用广度优先搜索算法(BFS)来寻找从起点到终点的最短路径。具体实现如下:
```
int[][] dirs = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}}; // 上下左右四个方向
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startRow, startCol}); // 将起点加入队列
while (!queue.isEmpty()) {
int[] curr = queue.poll();
int row = curr[0];
int col = curr[1];
if (row == endRow && col == endCol) { // 找到终点,退出循环
break;
}
for (int[] dir : dirs) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < numRows && newCol >= 0 && newCol < numCols && maze[newRow][newCol] == 0 && !visited[newRow][newCol]) {
queue.offer(new int[]{newRow, newCol}); // 将相邻的通路加入队列
visited[newRow][newCol] = true; // 标记为已访问
prev[newRow][newCol] = curr; // 记录前一个位置
}
}
}
```
最后,我们可以使用一个Stack来记录从终点到起点的路径,并在界面上展示出来。
```
Stack<int[]> stack = new Stack<>();
int[] curr = new int[]{endRow, endCol};
while (curr != null) {
stack.push(curr);
curr = prev[curr[0]][curr[1]];
}
while (!stack.isEmpty()) {
int[] pos = stack.pop();
Rectangle rect = new Rectangle(50, 50, Color.GREEN);
gridPane.add(rect, pos[1], pos[0]);
}
```
完整的代码如下:
```
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.GridPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Stack;
public class MazeGame extends Application {
private int[][] maze = {
{1, 1, 1, 1, 1},
{1, 0, 0, 0, 1},
{1, 0, 1, 0, 1},
{1, 0, 0, 0, 1},
{1, 1, 1, 1, 1}
};
private int numRows = maze.length;
private int numCols = maze[0].length;
private boolean[][] visited = new boolean[numRows][numCols];
private int[][] prev = new int[numRows][numCols];
private int startRow = 1;
private int startCol = 1;
private int endRow = 3;
private int endCol = 3;
@Override
public void start(Stage primaryStage) throws Exception {
GridPane gridPane = new GridPane();
for (int i = 0; i < maze.length; i++) {
for (int j = 0; j < maze[0].length; j++) {
Rectangle rect = new Rectangle(50, 50);
if (maze[i][j] == 1) {
rect.setFill(Color.BLACK);
} else {
rect.setFill(Color.WHITE);
}
gridPane.add(rect, j, i);
}
}
int[][] dirs = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startRow, startCol});
while (!queue.isEmpty()) {
int[] curr = queue.poll();
int row = curr[0];
int col = curr[1];
if (row == endRow && col == endCol) {
break;
}
for (int[] dir : dirs) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < numRows && newCol >= 0 && newCol < numCols && maze[newRow][newCol] == 0 && !visited[newRow][newCol]) {
queue.offer(new int[]{newRow, newCol});
visited[newRow][newCol] = true;
prev[newRow][newCol] = curr;
}
}
}
Stack<int[]> stack = new Stack<>();
int[] curr = new int[]{endRow, endCol};
while (curr != null) {
stack.push(curr);
curr = prev[curr[0]][curr[1]];
}
while (!stack.isEmpty()) {
int[] pos = stack.pop();
Rectangle rect = new Rectangle(50, 50, Color.GREEN);
gridPane.add(rect, pos[1], pos[0]);
}
Scene scene = new Scene(gridPane);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
用javafx写一个迷宫游戏要求有自动寻路功能并且可以调节迷宫难度
好的,下面是一个基于JavaFX的迷宫游戏的实现,包括自动寻路功能和难度调节。代码如下:
MazeGame.java:
```java
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import java.util.*;
public class MazeGame extends Application {
private static final int WIDTH = 500;
private static final int HEIGHT = 500;
private static final int EASY_SIZE = 10;
private static final int MEDIUM_SIZE = 20;
private static final int HARD_SIZE = 30;
private static final int CELL_SIZE = 20;
private static final Color WALL_COLOR = Color.BLACK;
private static final Color PATH_COLOR = Color.WHITE;
private static final Color START_COLOR = Color.GREEN;
private static final Color END_COLOR = Color.RED;
private static final Color VISITED_COLOR = Color.GRAY;
private static final int[][] DIRECTIONS = {{1, 0}, {-1, 0}, {0, 1}, {0, -1}};
private int[][] maze;
private boolean[][] visited;
private int startX, startY;
private int endX, endY;
private StackPane[][] cells;
private Button easyButton, mediumButton, hardButton, solveButton;
private Label messageLabel;
private boolean solving;
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
GridPane mazePane = new GridPane();
mazePane.setAlignment(Pos.CENTER);
root.setCenter(mazePane);
easyButton = new Button("Easy");
mediumButton = new Button("Medium");
hardButton = new Button("Hard");
solveButton = new Button("Solve");
easyButton.setOnAction(event -> generateMaze(EASY_SIZE));
mediumButton.setOnAction(event -> generateMaze(MEDIUM_SIZE));
hardButton.setOnAction(event -> generateMaze(HARD_SIZE));
solveButton.setOnAction(event -> solveMaze());
messageLabel = new Label("");
messageLabel.setAlignment(Pos.CENTER);
messageLabel.setPrefWidth(WIDTH);
BorderPane bottomPane = new BorderPane();
bottomPane.setLeft(easyButton);
bottomPane.setCenter(mediumButton);
bottomPane.setRight(hardButton);
bottomPane.setBottom(solveButton);
root.setTop(messageLabel);
root.setBottom(bottomPane);
primaryStage.setTitle("Maze Game");
primaryStage.setScene(new Scene(root, WIDTH, HEIGHT));
primaryStage.show();
generateMaze(EASY_SIZE);
}
private void generateMaze(int size) {
maze = new int[size][size];
visited = new boolean[size][size];
cells = new StackPane[size][size];
startX = (int) (Math.random() * size);
startY = (int) (Math.random() * size);
endX = (int) (Math.random() * size);
endY = (int) (Math.random() * size);
while (endX == startX && endY == startY) {
endX = (int) (Math.random() * size);
endY = (int) (Math.random() * size);
}
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if (i == startX && j == startY) {
maze[i][j] = 0;
} else if (i == endX && j == endY) {
maze[i][j] = 0;
} else {
maze[i][j] = 1;
}
visited[i][j] = false;
StackPane cell = new StackPane();
Rectangle rect = new Rectangle(CELL_SIZE, CELL_SIZE);
rect.setFill(WALL_COLOR);
cell.getChildren().add(rect);
cells[i][j] = cell;
GridPane.setConstraints(cell, j, i);
mazePane.getChildren().add(cell);
}
}
generateMazeRecursive(startX, startY);
solving = false;
messageLabel.setText("");
}
private void generateMazeRecursive(int x, int y) {
visited[x][y] = true;
List<int[]> neighbors = new ArrayList<>();
for (int[] dir : DIRECTIONS) {
int nx = x + dir[0];
int ny = y + dir[1];
if (nx >= 0 && nx < maze.length && ny >= 0 && ny < maze.length && !visited[nx][ny]) {
neighbors.add(new int[]{nx, ny});
}
}
while (!neighbors.isEmpty()) {
int idx = (int) (Math.random() * neighbors.size());
int[] neighbor = neighbors.get(idx);
int nx = neighbor[0];
int ny = neighbor[1];
if (maze[nx][ny] == 1) {
maze[nx][ny] = 0;
StackPane cell = cells[nx][ny];
cell.getChildren().clear();
cell.getChildren().add(new Rectangle(CELL_SIZE, CELL_SIZE, PATH_COLOR));
generateMazeRecursive(nx, ny);
}
neighbors.remove(idx);
}
}
private void solveMaze() {
if (solving) {
return;
}
solving = true;
messageLabel.setText("Solving...");
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startX, startY});
while (!queue.isEmpty()) {
int[] cur = queue.poll();
int x = cur[0];
int y = cur[1];
if (x == endX && y == endY) {
break;
}
for (int[] dir : DIRECTIONS) {
int nx = x + dir[0];
int ny = y + dir[1];
if (nx >= 0 && nx < maze.length && ny >= 0 && ny < maze.length && maze[nx][ny] == 0 && !visited[nx][ny]) {
queue.offer(new int[]{nx, ny});
visited[nx][ny] = true;
StackPane cell = cells[nx][ny];
cell.getChildren().clear();
cell.getChildren().add(new Rectangle(CELL_SIZE, CELL_SIZE, VISITED_COLOR));
}
}
}
if (visited[endX][endY]) {
messageLabel.setText("Path found!");
drawPath();
} else {
messageLabel.setText("No path found.");
}
solving = false;
}
private void drawPath() {
int x = endX;
int y = endY;
Stack<int[]> stack = new Stack<>();
while (x != startX || y != startY) {
stack.push(new int[]{x, y});
for (int[] dir : DIRECTIONS) {
int nx = x + dir[0];
int ny = y + dir[1];
if (nx >= 0 && nx < maze.length && ny >= 0 && ny < maze.length && visited[nx][ny] && maze[nx][ny] == 0) {
x = nx;
y = ny;
break;
}
}
}
stack.push(new int[]{startX, startY});
while (!stack.isEmpty()) {
int[] pos = stack.pop();
int px = pos[0];
int py = pos[1];
StackPane cell = cells[px][py];
cell.getChildren().clear();
cell.getChildren().add(new Rectangle(CELL_SIZE, CELL_SIZE, PATH_COLOR));
}
}
public static void main(String[] args) {
launch(args);
}
}
```
这个迷宫游戏包括四个按钮:Easy、Medium、Hard 和 Solve。Easy、Medium、Hard 分别对应不同的迷宫大小,点击后会自动生成迷宫。Solve 按钮用于寻找从起点到终点的路径。当点击 Solve 按钮时,程序会使用广度优先搜索算法遍历迷宫,找到从起点到终点的最短路径。
在实现中,迷宫是用一个二维数组来表示的,其中 0 表示路径,1 表示墙。生成迷宫时,程序使用递归深度优先搜索算法,从起点开始逐步拓展迷宫,直到达到终点。在搜索过程中,程序会将路径用白色矩形表示,墙用黑色矩形表示。在搜索完成后,程序会使用广度优先搜索算法遍历迷宫,找到从起点到终点的最短路径,并用灰色矩形表示已经访问过的节点,用白色矩形表示路径。
阅读全文
相关推荐
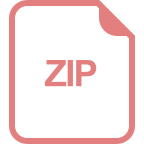

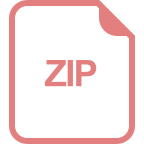
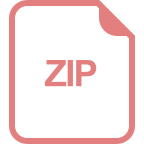
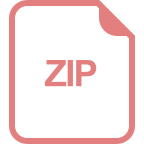
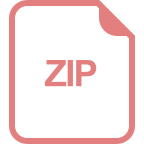
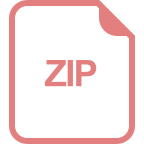
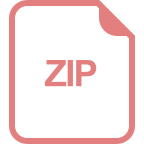
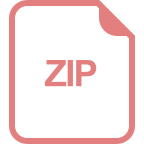
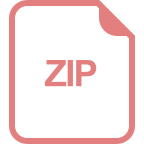
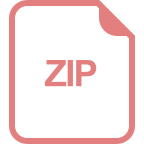
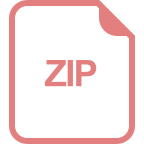
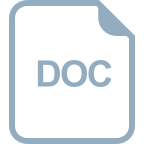
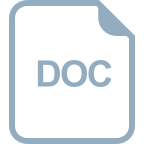
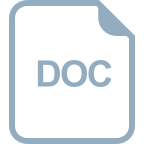