请用Python编写一个中考倒计时
时间: 2023-08-31 19:30:12 浏览: 139
### 回答1:
很高兴为您服务。这是一个用Python编写的中考倒计时的示例代码:# 导入需要的模块
import time# 定义中考日期
exam_date = "2020-07-07"# 计算当前日期与中考日期之间的天数
days_left = (time.strptime(exam_date, "%Y-%m-%d") - time.localtime()) // 86400# 输出中考倒计时
print("距离中考还有{}天!".format(days_left))
### 回答2:
在Python中编写一个中考倒计时可以使用datetime模块和time模块来完成。下面是一个简单的代码示例:
```python
import datetime
import time
def countdown(target_date):
while True:
current_datetime = datetime.datetime.now()
remaining_time = target_date - current_datetime
if remaining_time.total_seconds() <= 0:
print("中考已经开始!")
break
days = remaining_time.days
hours, remainder = divmod(remaining_time.seconds, 3600)
minutes, seconds = divmod(remainder, 60)
countdown_str = f"距离中考还有 {days} 天 {hours:02d} 小时 {minutes:02d} 分 {seconds:02d} 秒"
print(countdown_str, end="\r")
time.sleep(1)
# 设置中考日期
target_date = datetime.datetime(2022, 6, 1, 9, 0, 0)
# 调用倒计时函数
countdown(target_date)
```
这段代码使用了`countdown`函数来实现倒计时,并且通过循环和睡眠来实现每隔一秒钟更新一次倒计时显示。你可以根据需要修改`target_date`变量来设置你的中考日期。
运行这段代码后,程序会输出每秒钟更新一次的倒计时信息,直到中考日期到达,然后输出"中考已经开始!"。
### 回答3:
可以使用Python编写一个中考倒计时程序,具体如下:
```python
import datetime
def countdown(year, month, day):
exam_date = datetime.datetime(year, month, day)
today = datetime.datetime.now()
remaining_time = exam_date - today
days = remaining_time.days
hours = remaining_time.seconds // 3600
minutes = (remaining_time.seconds // 60) % 60
seconds = remaining_time.seconds % 60
print("距离中考还有:{}天 {}小时 {}分钟 {}秒".format(days, hours, minutes, seconds))
exam_year = int(input("请输入中考年份:"))
exam_month = int(input("请输入中考月份:"))
exam_day = int(input("请输入中考日期:"))
countdown(exam_year, exam_month, exam_day)
```
以上程序接受用户输入中考的年份、月份和日期,然后计算当前日期与中考日期之间的倒计时,输出距离中考还有多少天、小时、分钟和秒。
相关推荐
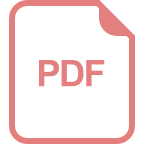
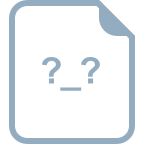
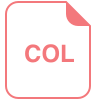
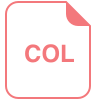
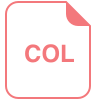
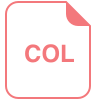
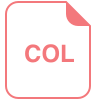









