p、v原语的模拟实现c++代码
时间: 2024-05-13 12:19:07 浏览: 83
以下是一个简单的C++代码实现p、v原语:
```c++
#include <pthread.h>
#include <semaphore.h>
#include <iostream>
using namespace std;
// 定义信号量和共享资源
sem_t sem;
int shared_resource = 0;
// 定义线程函数
void* producer(void* arg) {
while (true) {
sem_wait(&sem); // P操作
shared_resource++; // 生产者生产资源
cout << "Producer produces resource " << shared_resource << endl;
sem_post(&sem); // V操作
sleep(1);
}
pthread_exit(NULL);
}
void* consumer(void* arg) {
while (true) {
sem_wait(&sem); // P操作
if (shared_resource > 0) {
shared_resource--; // 消费者消耗资源
cout << "Consumer consumes resource " << shared_resource << endl;
}
sem_post(&sem); // V操作
sleep(2);
}
pthread_exit(NULL);
}
int main() {
pthread_t producer_thread, consumer_thread;
sem_init(&sem, 0, 1); // 初始化信号量
// 创建线程
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
// 等待线程结束
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
sem_destroy(&sem); // 销毁信号量
return 0;
}
```
注意,这只是一个简单的模拟实现,实际上p、v操作的实现需要更加复杂和精细,需要考虑到多线程的同步和互斥等问题。
阅读全文
相关推荐
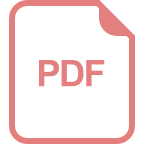

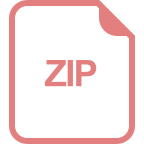
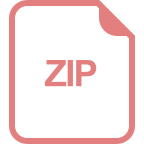
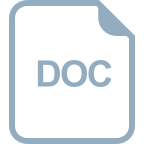
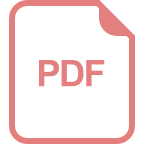
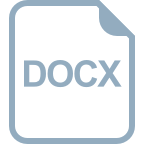
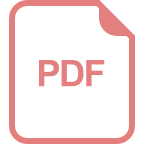
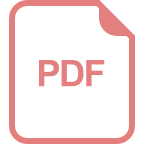
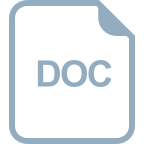
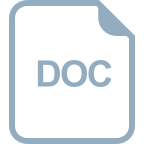
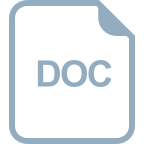
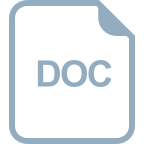
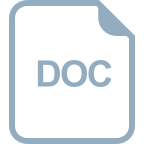
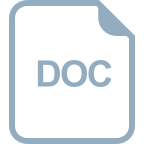
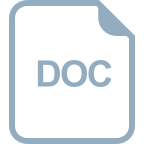
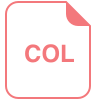