react mui box 如何实现loading效果
时间: 2024-05-04 11:20:00 浏览: 13
可以使用 MUI 的 CircularProgress 组件来实现 loading 效果。具体实现步骤如下:
1. 导入 CircularProgress 组件:
```javascript
import CircularProgress from '@mui/material/CircularProgress';
```
2. 在需要展示 loading 的地方,使用 CircularProgress 组件,并设置相应的 props:
```javascript
<Box sx={{ display: 'flex', flexDirection: 'column', alignItems: 'center' }}>
<CircularProgress size={80} />
<Typography variant="h6" sx={{ mt: 2 }}>
Loading...
</Typography>
</Box>
```
其中,设置了 CircularProgress 的 size 属性为 80,表示 loading 动画的大小为 80px;Typography 组件用于展示 "Loading..." 的文本。
3. 如果需要在 loading 时隐藏其他内容,可以使用 MUI 的 Box 组件的 visibility 属性来实现:
```javascript
<Box sx={{ visibility: isLoading ? 'hidden' : 'visible' }}>
{/* 这里放需要展示的内容 */}
</Box>
```
其中,isLoading 表示 loading 的状态,如果为 true,则隐藏 Box 中的内容;如果为 false,则展示 Box 中的内容。
完整示例代码如下:
```javascript
import CircularProgress from '@mui/material/CircularProgress';
import Box from '@mui/material/Box';
import Typography from '@mui/material/Typography';
function MyComponent() {
const [isLoading, setLoading] = useState(false);
// 模拟 loading 状态的变化
useEffect(() => {
setLoading(true);
setTimeout(() => {
setLoading(false);
}, 3000);
}, []);
return (
<Box sx={{ display: 'flex', flexDirection: 'column', alignItems: 'center' }}>
{isLoading && (
<Box sx={{ position: 'absolute', top: 0, left: 0, width: '100%', height: '100%', backgroundColor: 'rgba(255, 255, 255, 0.5)', zIndex: 1 }}>
<Box sx={{ display: 'flex', flexDirection: 'column', alignItems: 'center', justifyContent: 'center', position: 'absolute', top: '50%', left: '50%', transform: 'translate(-50%, -50%)' }}>
<CircularProgress size={80} />
<Typography variant="h6" sx={{ mt: 2 }}>
Loading...
</Typography>
</Box>
</Box>
)}
<Box sx={{ visibility: isLoading ? 'hidden' : 'visible' }}>
{/* 这里放需要展示的内容 */}
</Box>
</Box>
);
}
```
上述代码中还包含了一个定位在最上层的半透明层,用于遮盖其他内容,以达到更好的 loading 效果。
相关推荐
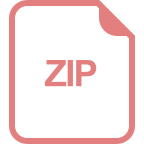
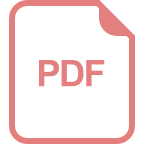














