C++向cmd里写入与读取
时间: 2024-11-03 22:17:23 浏览: 21
在C++中,与Windows命令提示符(CMD)交互通常涉及到文件流操作,特别是标准输入输出(stdin和stdout)。以下是基本的操作步骤:
**写入到CMD**(输出):
```cpp
#include <iostream>
#include <fstream>
int main() {
std::ofstream outfile("command.txt"); // 创建一个输出文件流
if (outfile.is_open()) { // 检查是否成功打开
outfile << "Command to be executed in CMD, e.g., 'dir'\n"; // 写入命令
outfile.close(); // 关闭文件
std::cout << "Command written to command.txt successfully.\n";
} else {
std::cerr << "Failed to open file.\n";
}
return 0;
}
```
在这个例子中,程序将一行文本写入`command.txt`文件,然后命令提示符可以运行这个文件来执行相应的命令。
**从CMD读取**(输入):
```cpp
#include <iostream>
#include <string>
#include <cstdlib> // 使用系统调用
std::string getCmdOutput(const char* cmd) {
std::stringstream ss;
FILE *pipe = popen(cmd, "r"); // 执行命令并连接到管道
if (!pipe) {
throw std::runtime_error("Error opening pipe.");
}
char buffer[128];
while (fgets(buffer, sizeof(buffer), pipe)) {
ss << buffer; // 将读取到的内容追加到字符串流
}
pclose(pipe); // 关闭管道
return ss.str();
}
int main() {
try {
std::string output = getCmdOutput("cmd.exe /c dir"); // 假设你想要执行的是目录查看命令
std::cout << "CMD output: " << output << "\n";
} catch (const std::exception& e) {
std::cerr << "Error executing command: " << e.what() << "\n";
}
return 0;
}
```
这里通过`popen`函数执行系统命令,并使用`fgets`逐行读取结果到字符串中。
阅读全文
相关推荐
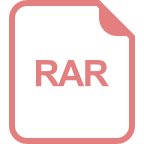
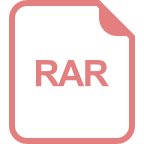
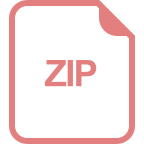
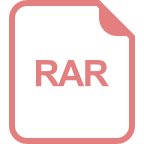
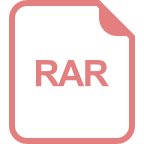
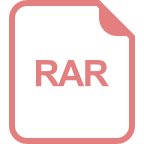
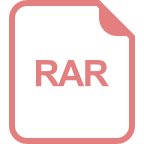
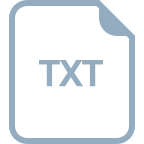
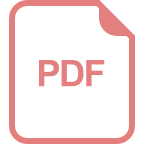
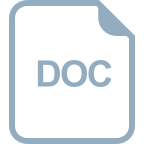
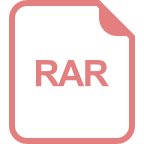
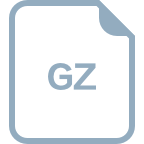
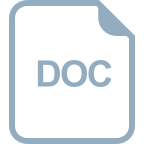
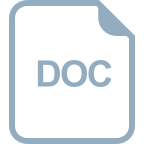
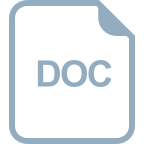
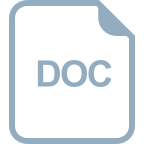


