如何用python来实现对FDDB数据集的评估。
时间: 2024-05-06 18:19:10 浏览: 12
FDDB(Face Detection Data Set and Benchmark)是一个人脸检测数据集,其中包含了来自多个图像数据集的5,171个人脸实例,其标注方式是用椭圆框出人脸区域。以下是用Python实现对FDDB数据集的评估的步骤:
1. 下载FDDB数据集:可以从官网上下载FDDB数据集。
2. 下载评估工具:FDDB提供了评估工具,可以从官网上下载。
3. 编写评估脚本:可以使用Python编写评估脚本,其中需要用到PIL(Python Imaging Library)库来读取图像和椭圆参数,用到numpy库来进行计算。
4. 运行评估脚本:运行评估脚本,输出评估结果。
以下是一个简单的评估脚本示例:
```python
import os
from PIL import Image
import numpy as np
# 读取FDDB数据集中的椭圆参数
def read_ellipse(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
num_faces = int(lines[0])
ellipses = []
for i in range(num_faces):
ellipse = [float(x) for x in lines[i+1].strip().split()]
ellipses.append(ellipse)
return np.array(ellipses)
# 读取人脸检测结果
def read_detection_result(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
num_faces = int(lines[0])
detections = []
for i in range(num_faces):
detection = [float(x) for x in lines[i+1].strip().split()]
detections.append(detection)
return np.array(detections)
# 计算重叠率
def calc_overlap(e1, e2):
a1, b1, c1, d1, e1, f1, _ = e1
a2, b2, c2, d2, e2, f2, _ = e2
cos_sq = np.cos(e1 - e2) ** 2
sin_sq = np.sin(e1 - e2) ** 2
alpha_sq = (a1 * np.sin(e1)) ** 2 + (b1 * np.cos(e1)) ** 2
beta_sq = (c1 * np.sin(e1)) ** 2 + (d1 * np.cos(e1)) ** 2
gamma_sq = (a2 * np.sin(e2)) ** 2 + (b2 * np.cos(e2)) ** 2
delta_sq = (c2 * np.sin(e2)) ** 2 + (d2 * np.cos(e2)) ** 2
numerator = (a1 * b1 - c1 * d1) * (a2 * b2 - c2 * d2)
denominator = np.sqrt(alpha_sq * beta_sq * gamma_sq * delta_sq)
return numerator / denominator * np.sqrt(cos_sq * alpha_sq + sin_sq * beta_sq)
# 评估函数
def evaluate(det_path, gt_path, iou_threshold=0.5):
det_files = os.listdir(det_path)
total_true_positives = 0
total_false_positives = 0
total_gt_faces = 0
for det_file in det_files:
# 读取检测结果
detection_result = read_detection_result(os.path.join(det_path, det_file))
# 读取对应的图像
img_file = det_file[:-4] + ".jpg"
img_path = os.path.join(gt_path, img_file)
img = Image.open(img_path)
# 读取标注的椭圆参数
ellipse_params = read_ellipse(os.path.join(gt_path, det_file[:-4] + ".txt"))
num_gt_faces = len(ellipse_params)
total_gt_faces += num_gt_faces
if num_gt_faces == 0:
continue
# 计算重叠率
overlaps = np.zeros((len(detection_result), num_gt_faces))
for i in range(len(detection_result)):
for j in range(num_gt_faces):
overlaps[i, j] = calc_overlap(detection_result[i], ellipse_params[j])
# 将每个检测结果与最大重叠率大于阈值的标注匹配
true_positives = np.zeros(len(detection_result))
false_positives = np.zeros(len(detection_result))
for j in range(num_gt_faces):
idx = np.argmax(overlaps[:, j])
if overlaps[idx, j] > iou_threshold:
true_positives[idx] = 1
else:
false_positives[idx] = 1
total_true_positives += np.sum(true_positives)
total_false_positives += np.sum(false_positives)
# 计算准确率和召回率
precision = total_true_positives / (total_true_positives + total_false_positives)
recall = total_true_positives / total_gt_faces
# 输出评估结果
print("Precision: {:.3f}".format(precision))
print("Recall: {:.3f}".format(recall))
```
使用以上代码,可以通过以下方式评估FDDB数据集中的人脸检测结果:
```python
evaluate(det_path='path/to/detection_results', gt_path='path/to/FDDB')
```
相关推荐
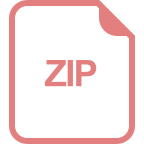
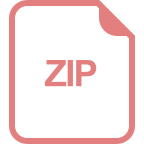













