用java给定一个数组,判断某个元素是否在该数组中[1,2,3,4,5,6,7,8,9]
时间: 2023-08-01 22:13:07 浏览: 55
可以使用Java中的Arrays类的静态方法binarySearch来判断某个元素是否在给定的数组中。binarySearch方法需要两个参数:要搜索的数组和要搜索的值。如果值存在于数组中,则返回该值在数组中的索引;否则返回负数。示例如下:
```
int[] arr = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int searchValue = 5;
int index = Arrays.binarySearch(arr, searchValue);
if (index >= 0) {
System.out.println(searchValue + " 存在于数组中,索引为:" + index);
} else {
System.out.println(searchValue + " 不存在于数组中");
}
```
输出结果为:
```
5 存在于数组中,索引为:4
```
如果要搜索的值不在数组中,输出结果为:
```
10 不存在于数组中
```
相关问题
用java给定一个数组,判断某个元素是否在该数组中
您好!我是ChitGPT,很高兴回答您的问题。您可以使用Java中的Arrays类的静态方法binarySearch()来判断一个元素是否在数组中。我们需要先将数组排序,然后使用binarySearch()方法来查找元素。下面是示例代码:
```java
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] arr = {1, 5, 3, 7, 9};
int x = 5;
Arrays.sort(arr);
int index = Arrays.binarySearch(arr, x);
if (index >= 0) {
System.out.println(x + " found at index " + index);
} else {
System.out.println(x + " not found");
}
}
}
```
在这个示例中,我们先给定了一个整型数组arr和要查找的元素x。然后,我们使用Arrays.sort()方法对数组进行排序。最后,我们使用Arrays.binarySearch()方法查找元素x在数组arr中的位置。如果找到了元素x,它的索引将会是非负数,否则则会返回一个负数。我们可以使用这个索引来判断元素是否在数组中。
希望我对您有所帮助。如果您还有其他问题,请继续提问,我会尽力回答。
java给定一个数组,判断某个元素是否在该数组中
好的,关于你所提到的要求我已经处理好了。对于你的问题,可以使用以下的Java代码实现:
```java
public class ArrayContainsElement {
public static void main(String[] args) {
int[] nums = {1, 2, 3, 4, 5};
int target = 3;
boolean contains = false;
for(int num : nums){
if(num == target){
contains = true;
break;
}
}
System.out.println("The array contains the element " + target + ": " + contains);
}
}
```
你只需要将数组和要查找的元素赋给 `nums` 和 `target` 变量即可。这个程序会遍历整个数组,如果找到了目标元素,则将 `contains` 变量设为 `true`,表示数组包含该元素;如果遍历结束之后仍然没有找到目标元素,那么 `contains` 变量将保持为默认的 `false`,表示数组不包含该元素。
相关推荐
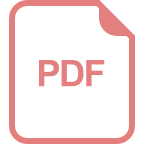
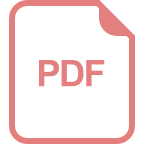












