matcher.group(2)怎么用c++实现
时间: 2023-08-26 07:09:44 浏览: 132
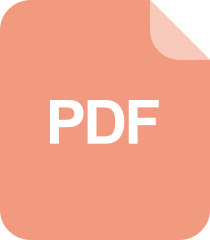
详解正则表达式Matcher类中group方法
`matcher.group(2)` 是 Java 语言中用于获取正则表达式匹配结果中第二个括号内的子串的方法。在 C++ 中,你可以使用正则表达式库 `std::regex` 来实现类似的功能。
以下是一个简单的示例代码:
```c++
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string s("Hello, World!");
std::regex r("(\\w+), (\\w+)!");
std::smatch m;
if (std::regex_search(s, m, r)) {
std::cout << m[2] << std::endl;
}
return 0;
}
```
在上面的代码中,我们定义了一个正则表达式 `r`,用于匹配字符串中以字母或数字组成的单词,并将第一个单词和第二个单词分别放在两组括号中。然后我们使用 `std::regex_search` 函数在字符串 `s` 中搜索匹配结果,并将结果存储在 `std::smatch` 对象 `m` 中。最后,我们通过 `m[2]` 获取第二个括号内的子串。
注意,C++ 中的正则表达式语法与 Java 略有不同,具体语法可以参考 C++ 标准库文档。
阅读全文
相关推荐
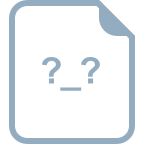
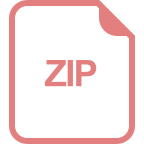



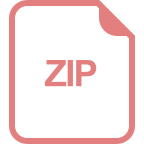
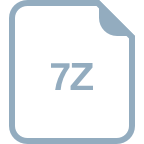
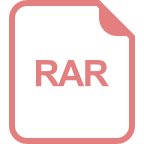
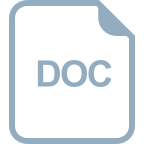
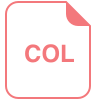
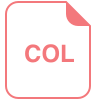

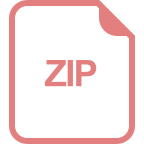
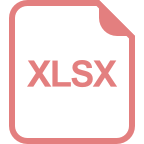
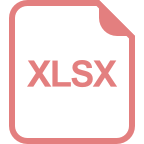
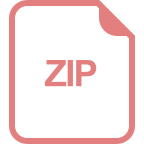