1. 如何判断一个一维信号服从高斯分布,用python编程实现。2. 讲解UNet并用pytorch实现一个UNet
时间: 2023-05-14 07:04:26 浏览: 193
1. 判断一维信号服从高斯分布的方法是通过绘制该信号的直方图并计算其均值和标准差,然后使用正态分布的概率密度函数计算该信号的概率密度值,如果该值接近于1,则可以认为该信号服从高斯分布。以下是用Python实现的代码:
import numpy as np
import matplotlib.pyplot as plt
from scipy.stats import norm
# 生成一维高斯分布信号
mu, sigma = 0, 0.1 # 均值和标准差
signal = np.random.normal(mu, sigma, 1000)
# 绘制信号的直方图
plt.hist(signal, bins=50, density=True, alpha=0.6, color='g')
# 计算信号的均值和标准差
mean = np.mean(signal)
std = np.std(signal)
# 计算信号的概率密度值
x = np.linspace(-0.5, 0.5, 100)
pdf = norm.pdf(x, mean, std)
# 绘制信号的概率密度函数
plt.plot(x, pdf, 'r', linewidth=2)
# 显示图像
plt.show()
2. UNet是一种用于图像分割的深度学习模型,其结构类似于自编码器,但在编码器和解码器之间添加了跨层连接,以保留更多的空间信息。以下是用PyTorch实现UNet的代码:
import torch
import torch.nn as nn
class DoubleConv(nn.Module):
def __init__(self, in_channels, out_channels):
super(DoubleConv, self).__init__()
self.conv = nn.Sequential(
nn.Conv2d(in_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True),
nn.Conv2d(out_channels, out_channels, kernel_size=3, padding=1),
nn.BatchNorm2d(out_channels),
nn.ReLU(inplace=True)
)
def forward(self, x):
return self.conv(x)
class UNet(nn.Module):
def __init__(self, in_channels=3, out_channels=1, features=[64, 128, 256, 512]):
super(UNet, self).__init__()
self.ups = nn.ModuleList()
self.downs = nn.ModuleList()
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
# 编码器部分
for feature in features:
self.downs.append(DoubleConv(in_channels, feature))
in_channels = feature
# 解码器部分
for feature in reversed(features):
self.ups.append(nn.ConvTranspose2d(feature*2, feature, kernel_size=2, stride=2))
self.ups.append(DoubleConv(feature*2, feature))
self.bottleneck = DoubleConv(features[-1], features[-1]*2)
self.final_conv = nn.Conv2d(features[0], out_channels, kernel_size=1)
def forward(self, x):
skip_connections = []
for down in self.downs:
x = down(x)
skip_connections.append(x)
x = self.pool(x)
x = self.bottleneck(x)
skip_connections = skip_connections[::-1]
for idx in range(0, len(self.ups), 2):
x = self.ups[idx](x)
skip_connection = skip_connections[idx//2]
if x.shape != skip_connection.shape:
x = nn.functional.interpolate(x, size=skip_connection.shape[2:], mode='bilinear', align_corners=True)
concat_skip = torch.cat((skip_connection, x), dim=1)
x = self.ups[idx+1](concat_skip)
return self.final_conv(x)
阅读全文
相关推荐
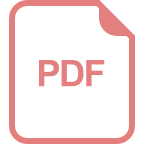
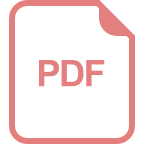
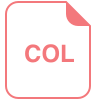
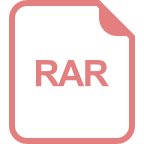
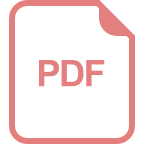
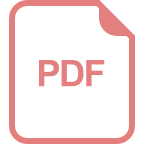
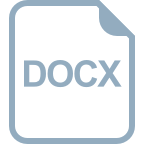
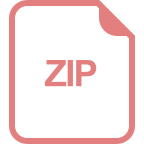
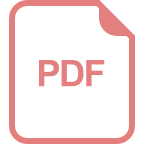
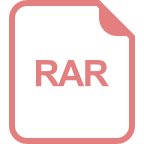
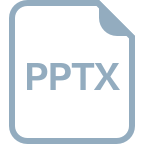
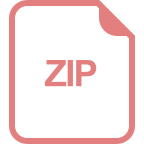
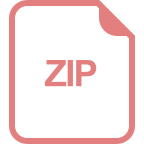
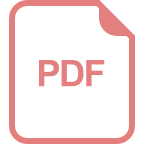
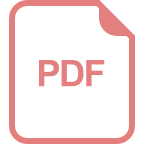
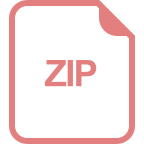