c++字符串的拼接
时间: 2024-12-10 22:18:33 浏览: 9
在C++中,字符串的拼接可以通过多种方法实现,主要包括使用`std::string`类的操作符重载、使用`std::string`类的成员函数以及使用C风格的字符串函数。以下是几种常见的字符串拼接方法:
### 1. 使用`std::string`类的操作符重载
`std::string`类重载了`+`和`+=`操作符,可以方便地进行字符串拼接。
```cpp
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
// 使用 + 操作符
std::string result = str1 + str2;
std::cout << result << std::endl; // 输出: Hello, World!
// 使用 += 操作符
str1 += str2;
std::cout << str1 << std::endl; // 输出: Hello, World!
return 0;
}
```
### 2. 使用`std::string`类的成员函数
`std::string`类提供了`append()`成员函数用于字符串拼接。
```cpp
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
// 使用 append() 成员函数
str1.append(str2);
std::cout << str1 << std::endl; // 输出: Hello, World!
return 0;
}
```
### 3. 使用C风格的字符串函数
在C++中,也可以使用C风格的字符串函数如`strcat()`和`strcpy()`进行字符串拼接。
```cpp
#include <iostream>
#include <cstring>
int main() {
char str1[20] = "Hello, ";
char str2[] = "World!";
// 使用 strcat() 函数
strcat(str1, str2);
std::cout << str1 << std::endl; // 输出: Hello, World!
return 0;
}
```
### 4. 使用`std::ostringstream`
`std::ostringstream`类可以用于更复杂的字符串拼接操作。
```cpp
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::ostringstream oss;
std::string str1 = "Hello, ";
std::string str2 = "World!";
// 使用 ostringstream 进行拼接
oss << str1 << str2;
std::string result = oss.str();
std::cout << result << std::endl; // 输出: Hello, World!
return 0;
}
```
通过以上几种方法,可以根据具体需求选择合适的字符串拼接方式。
阅读全文
相关推荐
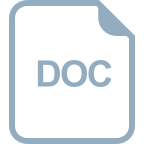
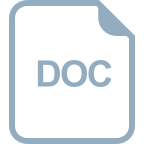
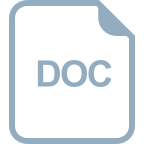
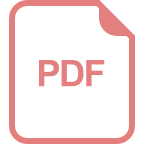
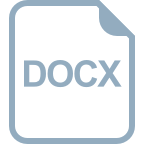
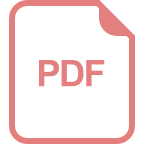
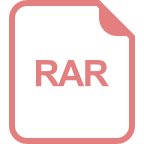
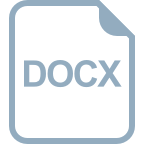
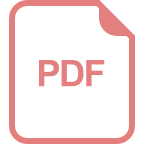
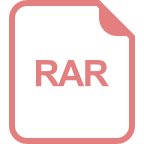
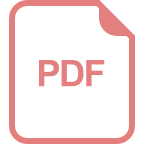
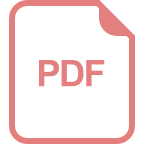
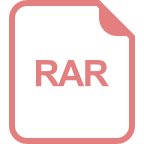
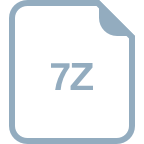
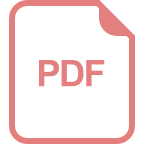