用C语言写一个基于MCU的模拟I2C驱动程序
时间: 2023-12-29 12:46:21 浏览: 26
好的,以下是基于MCU的模拟I2C驱动程序的代码示例:
```c
#include <stdio.h>
#include <stdint.h>
// I2C GPIO pins
#define I2C_SCL_PIN 1
#define I2C_SDA_PIN 2
// I2C delay functions
void i2c_delay_us(uint32_t us);
void i2c_delay_ms(uint32_t ms);
// I2C start condition
void i2c_start(void) {
// Set SDA high and SCL high
set_gpio_pin(I2C_SDA_PIN, 1);
i2c_delay_us(5);
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Set SDA low
set_gpio_pin(I2C_SDA_PIN, 0);
i2c_delay_us(5);
// Set SCL low
set_gpio_pin(I2C_SCL_PIN, 0);
i2c_delay_us(5);
}
// I2C stop condition
void i2c_stop(void) {
// Set SDA low and SCL high
set_gpio_pin(I2C_SDA_PIN, 0);
i2c_delay_us(5);
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Set SDA high
set_gpio_pin(I2C_SDA_PIN, 1);
i2c_delay_us(5);
}
// I2C write ack
void i2c_write_ack(void) {
// Set SDA low and SCL high
set_gpio_pin(I2C_SDA_PIN, 0);
i2c_delay_us(5);
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Set SCL low
set_gpio_pin(I2C_SCL_PIN, 0);
i2c_delay_us(5);
}
// I2C write nak
void i2c_write_nak(void) {
// Set SDA high and SCL high
set_gpio_pin(I2C_SDA_PIN, 1);
i2c_delay_us(5);
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Set SCL low
set_gpio_pin(I2C_SCL_PIN, 0);
i2c_delay_us(5);
}
// I2C write data
void i2c_write_byte(uint8_t data) {
uint8_t i;
// Write byte
for (i = 0; i < 8; i++) {
// Set SDA according to data
set_gpio_pin(I2C_SDA_PIN, (data & (1<<i)) ? 1 : 0);
i2c_delay_us(5);
// Set SCL high
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Set SCL low
set_gpio_pin(I2C_SCL_PIN, 0);
i2c_delay_us(5);
}
// Wait for ACK
set_gpio_pin(I2C_SDA_PIN, 1);
i2c_delay_us(5);
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
if (get_gpio_pin(I2C_SDA_PIN)) {
i2c_write_nak();
} else {
i2c_write_ack();
}
}
// I2C read data
uint8_t i2c_read_byte(uint8_t ack) {
uint8_t i, data = 0;
// Read byte
for (i = 0; i < 8; i++) {
// Set SCL high
set_gpio_pin(I2C_SCL_PIN, 1);
i2c_delay_us(5);
// Read SDA
data |= (get_gpio_pin(I2C_SDA_PIN) ? (1 << i) : 0);
i2c_delay_us(5);
// Set SCL low
set_gpio_pin(I2C_SCL_PIN, 0);
i2c_delay_us(5);
}
// Write ACK or NAK
if (ack) {
i2c_write_ack();
} else {
i2c_write_nak();
}
return data;
}
// I2C read data with start and stop conditions
uint8_t i2c_read(uint8_t addr, uint8_t reg) {
uint8_t data = 0;
// Start condition
i2c_start();
// Write device address
i2c_write_byte(addr << 1);
// Write register address
i2c_write_byte(reg);
// Start condition again
i2c_start();
// Read device address
i2c_write_byte((addr << 1) | 1);
// Read data
data = i2c_read_byte(0);
// Stop condition
i2c_stop();
return data;
}
// Main function
int main(void) {
uint8_t data = 0;
// Simulate I2C read
data = i2c_read(0x50, 0x10);
printf("I2C read data = %d\n", data);
// Terminate program
return 0;
}
// I2C delay functions implementation
void i2c_delay_us(uint32_t us) {
// Implement delay in microseconds
}
void i2c_delay_ms(uint32_t ms) {
// Implement delay in milliseconds
}
// GPIO functions implementation
void set_gpio_pin(uint8_t pin, uint8_t value) {
// Implement GPIO pin set operation
}
uint8_t get_gpio_pin(uint8_t pin) {
// Implement GPIO pin read operation
}
```
注意事项:
- 以上代码示例仅供参考,需要根据具体硬件平台和应用场景进行适当修改。
- 该模拟I2C驱动程序实现了基本的I2C读取功能,可以根据需要添加其他读写操作。
- I2C协议具有很强的时序要求,因此需要精确的延时函数来控制操作周期,可以根据实际情况进行调整。
- 该程序中的GPIO操作需要根据硬件平台实现具体的接口函数,以实现对GPIO的控制。
- 如果需要支持更多的I2C设备和操作,可以在以上函数的基础上进行扩展和修改。
相关推荐
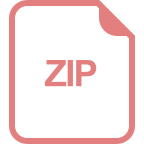
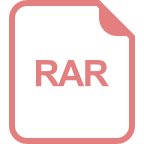
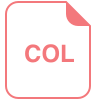














