用c语言算法随机生成10个整数,输出线性表,验证结果。
时间: 2024-09-29 13:15:25 浏览: 45
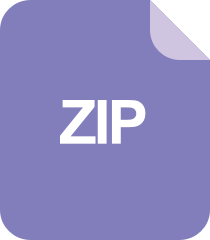
求链式线性表的倒数第K项_C语言_K._

为了在C语言中生成并输出随机整数,并构成一个简单的线性表,你可以使用`<time.h>`库获取当前时间作为种子生成随机数,然后将它们存储在一个数组中。以下是实现这一过程的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h> // 需要用到time()函数
#define SIZE 10 // 定义数组大小为10
// 生成随机整数的范围可以自定义,这里假设在1到100之间
#define MIN_VALUE 1
#define MAX_VALUE 100
int main() {
srand(time(0)); // 使用当前时间作为随机数生成器的种子
int numbers[SIZE]; // 存储随机数的数组
// 生成并填充数组
for (int i = 0; i < SIZE; ++i) {
numbers[i] = rand() % (MAX_VALUE - MIN_VALUE + 1) + MIN_VALUE; // 生成1到MAX_VALUE的随机数
}
// 输出线性表
printf("Randomly generated integers: ");
for (int i = 0; i < SIZE; ++i) {
printf("%d ", numbers[i]);
}
printf("\n");
// 验证结果:简单地检查数组是否包含了10个不同的数字即可
int unique_numbers = SIZE;
for (int i = 0; i < SIZE; ++i) {
for (int j = i+1; j < SIZE; ++j) {
if (numbers[i] == numbers[j]) {
unique_numbers--;
break;
}
}
}
if (unique_numbers == SIZE) {
printf("All elements are distinct, verification passed.\n");
} else {
printf("Some elements are repeated, verification failed.\n");
}
return 0;
}
```
运行此程序后,它会生成一个包含10个1到100之间的随机整数的列表,并检查所有元素是否唯一。如果所有数字都不同,那么验证就会通过。
阅读全文
相关推荐
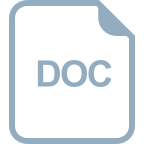
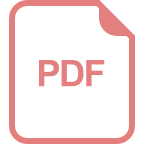

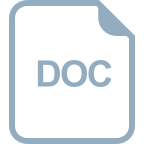
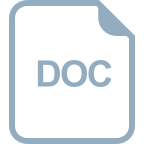












