python以UTF8编码把中文写入文件
时间: 2023-11-24 14:14:52 浏览: 35
在Python中,要以UTF-8编码将中文写入文件,可以使用以下代码:
```python
# 打开文件,指定编码格式为UTF-8
with open("file.txt", "w", encoding="utf-8") as f:
# 写入中文内容
f.write("你好,世界!")
```
其中,`open()` 函数用于打开文件,第一个参数是文件名,第二个参数是打开文件的模式,这里使用 "w" 表示写入模式。第三个参数 `encoding` 表示指定编码格式为UTF-8。
`with` 语句是一个上下文管理器,它可以自动帮助我们关闭文件,避免因为打开文件后忘记关闭而导致的问题。
在 `with` 语句块内,我们使用 `write()` 方法将中文内容写入文件。注意,在Python3中,字符串默认使用Unicode编码,因此如果在字符串前加上 "u" 前缀,就表示使用Unicode编码。
执行完以上代码后,就可以在当前目录下生成一个名为 "file.txt" 的文件,其中包含了 "你好,世界!" 这段中文内容。
相关问题
python把123以utf-8写入到本地txt文件
可以使用Python内置的 `open()` 函数和文件对象的 `write()` 方法来实现将字符串写入到本地txt文件中。示例代码如下:
```python
content = "123"
with open("test.txt", "w", encoding="utf-8") as f:
f.write(content)
```
其中,`"test.txt"` 是文件名,`"w"` 表示以写入模式打开文件,`encoding="utf-8"` 表示使用 utf-8 编码将字符串写入到文件中。在 `with` 语句中打开文件后,使用 `write()` 方法将字符串写入到文件中,最后自动关闭文件。
python生成csv文件并以utf-8编码保存
在 Python 中,可以使用 `csv` 模块来生成 CSV 文件,并使用 `encoding='utf-8'` 参数来指定编码为 UTF-8。以下是一个示例代码:
```python
import csv
# 要写入的数据
data = [
['姓名', '年龄', '性别'],
['张三', 18, '男'],
['李四', 20, '女'],
['王五', 22, '男']
]
# 打开文件并写入数据
with open('data.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
for row in data:
writer.writerow(row)
```
在上面的代码中,我们首先定义了要写入的数据,然后使用 `open()` 函数打开文件,并指定文件名为 `data.csv`,模式为写入模式 (`'w'`),`newline=''` 参数用于避免写入空行,`encoding='utf-8'` 参数指定编码为 UTF-8。
接着,我们创建一个 `csv.writer` 对象,并使用 `writerow()` 方法逐行写入数据。最后,使用 `with` 语句自动关闭文件。
相关推荐
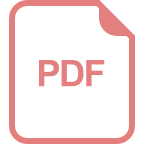
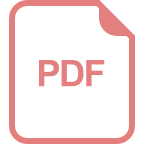
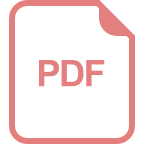












