convert sprintf(fmt, names(df)) to sprintf(fmt, names(df)[1], names(df)[2]) in a simple way R
时间: 2024-03-06 21:47:15 浏览: 83
You can use the `sprintf` function in combination with the `paste` function to achieve this in a simple way. Here's an example:
```
# Define the format string and the data frame
fmt <- "The first column is %s and the second column is %s"
df <- data.frame(a = 1:3, b = 4:6)
# Use sprintf and paste to create the new format string
new_fmt <- sprintf(fmt, paste(names(df)[1], names(df)[2], sep = ", "))
# Output the new format string
new_fmt
```
This will output:
```
[1] "The first column is a, the second column is b"
```
As you can see, the new format string now includes only the first and second column names of the data frame.
相关问题
improve the code as it gets error "Error in sprintf(fmt, names(df)) : too few arguments"
This error typically occurs when the number of placeholders in the `sprintf` format string does not match the number of arguments provided. To fix this error, you need to ensure that the number of placeholders in the `sprintf` format string matches the number of arguments being passed.
Here is an example of how you can fix this error:
```R
# Example data frame
df <- data.frame(col1 = c(1, 2, 3), col2 = c("A", "B", "C"))
# Incorrect usage of sprintf - produces error
fmt <- "Columns: %s, %s"
message(sprintf(fmt, names(df)))
# Correct usage of sprintf - fixes the error
fmt <- "Columns: %s, %s"
message(sprintf(fmt, names(df)[1], names(df)[2]))
```
In the above example, we first define a data frame `df`. We then define a format string `fmt` with two placeholders for column names. However, when we pass `names(df)` as arguments to `sprintf`, we get an error because we only provided one argument instead of two.
To fix the error, we need to pass two arguments to `sprintf` that correspond to the two placeholders in `fmt`. We can do this by indexing `names(df)` to get the column names for each placeholder.
fmt.sprintf
`fmt.Sprintf`是一个用于格式化字符串的函数,它返回一个格式化后的字符串。它的使用方法与`fmt.Printf`类似,但是不会将格式化后的字符串输出到标准输出流中,而是返回一个字符串。下面是一个使用`fmt.Sprintf`的例子:
```go
package main
import "fmt"
func main() {
name := "Alice"
age := 30
str := fmt.Sprintf("My name is %s and I'm %d years old.", name, age)
fmt.Println(str)
}
```
输出结果为:
```
My name is Alice and I'm 30 years old.
```
阅读全文
相关推荐
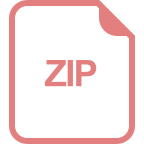
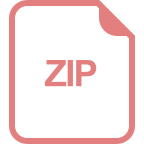
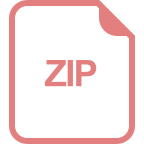













