设计函数实现将一个字符串中小写字母转化为大写字母。
时间: 2023-11-18 21:03:12 浏览: 230
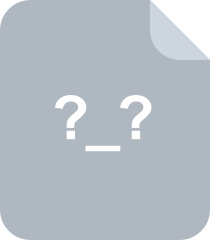
实现一个函数,把一个字符串中的字符从小写转为大写。

可以使用 Python 内置函数 `upper()` 将字符串中的小写字母转化为大写字母,具体实现如下:
```python
def to_uppercase(string):
return string.upper()
```
其中,`string.upper()` 返回将字符串 `string` 中所有小写字母转化为大写字母后的字符串。可以通过以下方式调用该函数:
```python
print(to_uppercase("Hello, world!")) # 输出 "HELLO, WORLD!"
```
需要注意的是,该函数不会修改原字符串,而是返回一个新的字符串。如果需要修改原字符串,可以使用 `string` 对象自身的 `upper()` 方法。
阅读全文
相关推荐


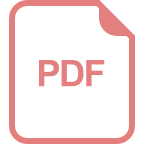
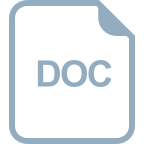










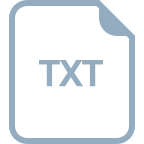