平衡二叉树操作的演示代码
时间: 2024-07-04 22:00:32 浏览: 98
平衡二叉树(如AVL树或红黑树)是一种特殊的二叉搜索树,它的每个节点的左右子树的高度差不超过1。这种数据结构保证了插入、删除和查找操作的时间复杂度为O(log n)。下面是一个简单的Python代码演示了AVL树的插入操作:
```python
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
self.height = 1
class AVLTree:
def insert(self, root, key):
# ... (AVL插入逻辑,包括旋转操作)
# 这里简化为基本插入,假设没有旋转操作
if not root:
return Node(key)
elif key < root.key:
root.left = self.insert(root.left, key)
else:
root.right = self.insert(root.right, key)
# Update the height of the current node
root.height = 1 + max(self.getHeight(root.left), self.getHeight(root.right))
# Get the balance factor
balance = self.getBalance(root)
# If the node is unbalanced, perform appropriate rotation(s)
# ... (这里省略了平衡调整部分)
return root
# ... (其他辅助方法,如getHeight()和getBalance())
# 示例使用
avl = AVLTree()
root = None
keys = [5, 3, 7, 1, 9, 2, 6, 8, 4]
for k in keys:
root = avl.insert(root, k)
# ... (展示插入后的平衡二叉树)
```
这个代码片段展示了如何使用AVL树的基本插入操作,但实际的实现会涉及到旋转操作(左旋、右旋)以保持树的平衡。
阅读全文
相关推荐
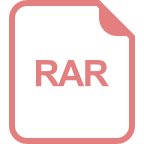
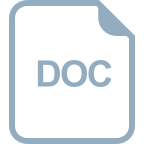
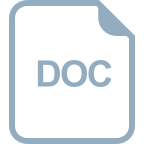
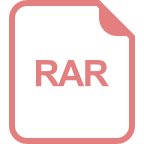
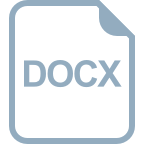
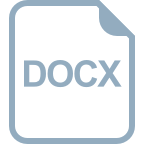
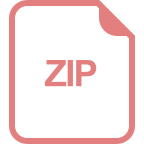
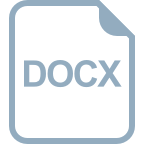
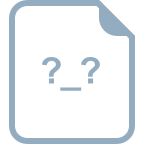
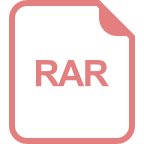
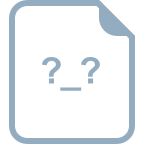
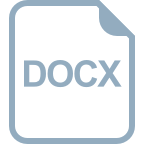
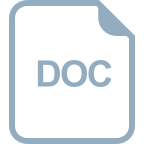
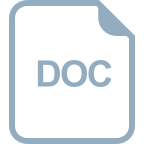