efcore 递归mysql树形结构
时间: 2023-06-30 16:20:09 浏览: 49
在使用 Entity Framework Core 操作 MySQL 数据库时,可以使用递归公用表表达式(CTE)来查询树形结构数据。下面是一个简单的示例:
假设我们有一个表 `category` 存储了商品分类的树形结构,表结构如下:
```csharp
public class Category
{
public int Id { get; set; }
public string Name { get; set; }
public int? ParentId { get; set; }
}
public class MyDbContext : DbContext
{
public DbSet<Category> Categories { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseMySQL("connection_string");
}
}
```
其中,`ParentId` 表示父分类的 ID,根节点的 `ParentId` 为 NULL。
现在我们需要查询某个分类的所有子分类,包括子分类的子分类,以此类推。可以使用递归公用表表达式(CTE)来实现递归查询,如下所示:
```csharp
using System.Linq;
using Microsoft.EntityFrameworkCore;
public static class CategoryExtensions
{
public static IQueryable<Category> GetDescendants(this IQueryable<Category> query, int id)
{
var rawSql = @"
WITH RECURSIVE cte AS (
SELECT id, name, parent_id
FROM category
WHERE id = {0}
UNION ALL
SELECT c.id, c.name, c.parent_id
FROM category c
JOIN cte ON c.parent_id = cte.id
)
SELECT id, name, parent_id FROM cte;";
return query.FromSqlRaw(rawSql, id);
}
}
```
上述代码定义了一个静态扩展方法 `GetDescendants`,用于查询指定分类 ID 的所有子分类。在方法中,我们使用了递归公用表表达式(CTE)查询树形结构数据。具体来说,我们首先定义了一个 CTE 名为 `cte`,以参数 `id` 作为起始分类的 ID。然后使用 UNION ALL 将递归查询的结果与分类表连接,直到没有更多子分类为止。最后,输出所有子分类的 ID、名称和父分类的 ID。
可以通过以下代码调用 `GetDescendants` 方法,并指定起始分类的 ID:
```csharp
using (var context = new MyDbContext())
{
var descendants = context.Categories.GetDescendants(1);
foreach (var descendant in descendants)
{
Console.WriteLine($"{descendant.Id} - {descendant.Name} - {descendant.ParentId}");
}
}
```
上述代码将输出所有子分类的 ID、名称和父分类的 ID。
相关推荐
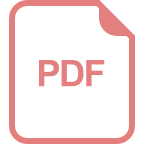













