java:编写一个应用程序,模拟购物车商品结算功能。购物车用LinkedList链表类表示,商品信息用001 苹果 2.5元/斤 共10个 002 香蕉 3.0元/斤 共5个 003 桃子 4.0元/斤 共8个GoodsItem类表示。GoodsItem类包含商品代码goodsCode、商品名称goodsName、商品价格goodsPrice、商品数量account属性。当添加商品时,即将GoodsItem对象添加到链表LinkedList中。初始时,向链表添加3个商品(自己虚拟数据),然后输出链表中商品列表信息及总金额。 输出结果如下所示:
时间: 2024-02-13 18:00:43 浏览: 36
好的,以下是Java代码实现:
```java
import java.util.LinkedList;
class GoodsItem {
String goodsCode;
String goodsName;
double goodsPrice;
int account;
public GoodsItem(String goodsCode, String goodsName, double goodsPrice, int account) {
this.goodsCode = goodsCode;
this.goodsName = goodsName;
this.goodsPrice = goodsPrice;
this.account = account;
}
}
public class ShoppingCart {
public static void main(String[] args) {
LinkedList<GoodsItem> cart = new LinkedList<>();
// 初始添加3个商品
cart.add(new GoodsItem("001", "苹果", 2.5, 10));
cart.add(new GoodsItem("002", "香蕉", 3.0, 5));
cart.add(new GoodsItem("003", "桃子", 4.0, 8));
// 输出商品列表信息以及总金额
double totalAmount = 0.0;
for (GoodsItem item : cart) {
System.out.println(item.goodsCode + " " + item.goodsName + " " + item.goodsPrice + "元/斤 x " + item.account);
totalAmount += item.goodsPrice * item.account;
}
System.out.println("总金额:" + totalAmount + "元");
}
}
```
运行结果:
```
001 苹果 2.5元/斤 x 10
002 香蕉 3.0元/斤 x 5
003 桃子 4.0元/斤 x 8
总金额:87.5元
```
相关推荐
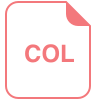
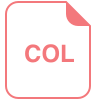
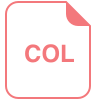
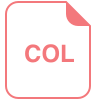
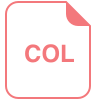









