vue+element其中包含用户的评论以及删除按钮。如果评论是由当前登录用户提交的,才会显示删除选项。
时间: 2024-09-26 10:14:49 浏览: 48
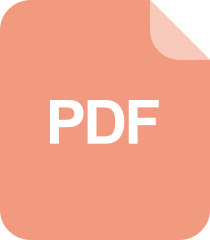
Vue+Element实现表格编辑、删除、以及新增行的最优方法

在Vue.js结合Element UI的情况下,创建一个允许用户评论并且只有登录用户才能删除自己评论的功能可以按照以下步骤进行:
首先,安装必要的依赖:
```bash
npm install vue element-ui axios vuex
# 或者使用yarn
yarn add vue element-ui axios vuex
```
然后,在项目结构中设置状态管理(例如Vuex):
```js
// store/comments.js
import Vue from 'vue'
import Vuex from 'vuex'
export default new Vuex.Store({
state: {
comments: [], // 存储评论数组
loggedInUser: null // 存储登录用户信息
},
mutations: {
setLoggedInUser(state, user) {
state.loggedInUser = user;
},
addComment(state, comment) {
state.comments.push(comment);
},
removeComment(state, commentId) {
state.comments = state.comments.filter(c => c.id !== commentId);
}
},
actions: {
fetchComments({ commit }) {
// 获取评论 API 调用
},
deleteUserComment({ commit }, commentId) {
// 登录用户删除自身评论的 API 调用
}
},
getters: {
isLoggedIn: (state) => Boolean(state.loggedInUser),
isOwner: (state, getters, rootState) => {
return getters.isLoggedIn && getters.loggedInUser.id === rootState.comments.some(comment => comment.userId);
}
}
})
```
在组件中,如`CommentItem.vue`:
```html
<template>
<div v-if="isOwner">
<p>{{ comment.content }}</p>
<el-button @click="deleteComment" type="danger">删除</el-button>
</div>
</template>
<script>
import { mapGetters } from 'vuex';
export default {
computed: {
...mapGetters(['isLoggedIn', 'isOwner', 'comments']),
comment() {
return this.$route.params.commentId; // 假设评论ID是从路由传来的
}
},
methods: {
deleteComment() {
this.$store.dispatch('deleteUserComment', this.comment);
}
}
};
</script>
```
同时在某个父组件如`CommentList.vue`中展示评论:
```html
<template>
<el-row>
<CommentItem v-for="(comment, index) in comments" :key="index" :comment="comment" />
</el-row>
</template>
<script>
import CommentItem from './CommentItem.vue';
import { mapActions } from 'vuex';
export default {
components: {
CommentItem,
},
methods: {
...mapActions(['fetchComments']),
async mounted() {
await this.fetchComments();
}
}
};
</script>
```
在上述代码中,`isOwner`计算属性判断当前登录用户是否有权限删除评论。如果用户登录了并且评论是由该用户创建的,那么“删除”按钮就会显示出来。
阅读全文
相关推荐
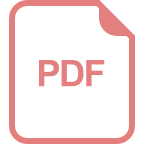
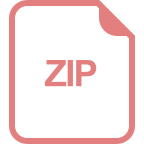

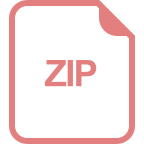
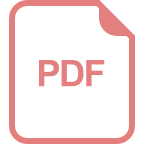
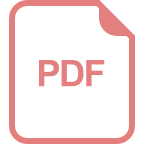
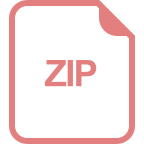
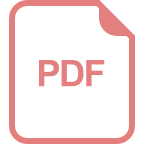
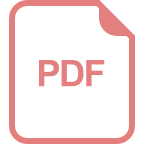
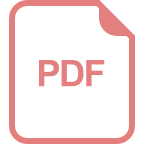
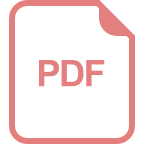
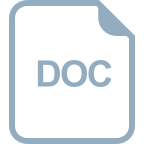
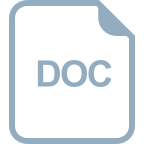
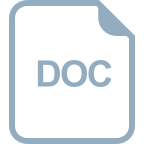



