用qcustomplot插件,写一段有选择框功能,选择框选中的地方,数据只拉伸x轴数据,y轴数据不动,并且y轴的范围也不变,只变x轴的范围,选框中的y轴数据也不变,要根据范围显示数值,只改变x轴数据
时间: 2023-09-10 10:08:59 浏览: 66
好的,以下是使用qcustomplot插件实现该功能的代码:
首先,我们需要在头文件中包含qcustomplot的头文件:
```cpp
#include "qcustomplot.h"
```
然后,在我们的QWidget中添加一个QCustomPlot控件:
```cpp
QCustomPlot *customPlot = new QCustomPlot(this);
```
接下来,我们需要设置我们的QCustomPlot控件的x轴和y轴范围:
```cpp
customPlot->xAxis->setRange(0, 10);
customPlot->yAxis->setRange(-1, 1);
```
然后,我们需要绘制一些数据点:
```cpp
QVector<double> x(101), y(101);
for (int i=0; i<101; ++i)
{
x[i] = i/50.0 - 1;
y[i] = qSin(x[i]*3.14);
}
customPlot->addGraph();
customPlot->graph(0)->setData(x, y);
```
现在,我们需要添加一个选择框。我们可以使用QCPItemRect来实现:
```cpp
QCPItemRect *selectionRect = new QCPItemRect(customPlot);
customPlot->addItem(selectionRect);
selectionRect->setVisible(false);
```
我们需要在我们的QCustomPlot控件上安装事件过滤器:
```cpp
customPlot->installEventFilter(this);
```
然后,我们需要在事件过滤器中处理鼠标点击和移动事件:
```cpp
bool MyWidget::eventFilter(QObject *obj, QEvent *event)
{
if (obj == customPlot && event->type() == QEvent::MouseButtonPress)
{
QMouseEvent *mouseEvent = static_cast<QMouseEvent*>(event);
if (mouseEvent->button() == Qt::LeftButton)
{
// clear any previous selection
selectionRect->setVisible(false);
selectionStart = customPlot->xAxis->pixelToCoord(mouseEvent->pos().x());
selectionRect->setRect(QRectF(selectionStart, customPlot->yAxis->range().lower, 0, customPlot->yAxis->range().size()));
selectionRect->setVisible(true);
return true;
}
}
else if (obj == customPlot && event->type() == QEvent::MouseMove)
{
QMouseEvent *mouseEvent = static_cast<QMouseEvent*>(event);
if (selectionRect->visible())
{
double currentX = customPlot->xAxis->pixelToCoord(mouseEvent->pos().x());
double width = currentX - selectionStart;
selectionRect->setRect(QRectF(selectionStart, customPlot->yAxis->range().lower, width, customPlot->yAxis->range().size()));
return true;
}
}
else if (obj == customPlot && event->type() == QEvent::MouseButtonRelease)
{
QMouseEvent *mouseEvent = static_cast<QMouseEvent*>(event);
if (mouseEvent->button() == Qt::LeftButton && selectionRect->visible())
{
double selectionEnd = customPlot->xAxis->pixelToCoord(mouseEvent->pos().x());
double selectionMin = qMin(selectionStart, selectionEnd);
double selectionMax = qMax(selectionStart, selectionEnd);
customPlot->xAxis->setRange(selectionMin, selectionMax);
selectionRect->setVisible(false);
return true;
}
}
return QWidget::eventFilter(obj, event);
}
```
最后,我们需要定义一个选择框的起点和一个QCPItemRect指针:
```cpp
double selectionStart;
QCPItemRect *selectionRect;
```
这样,我们就完成了使用qcustomplot插件实现选择框功能的代码。
阅读全文
相关推荐
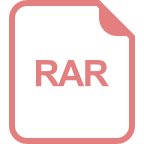



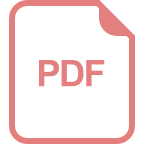
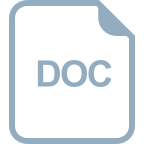
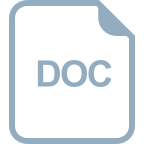
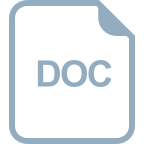
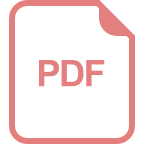
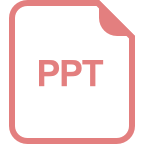
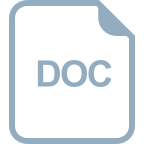
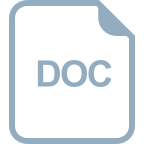
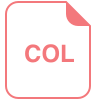
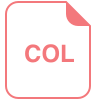
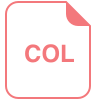
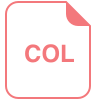
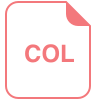