python将输入文本转换为txt保存到指定路径
时间: 2024-01-16 20:01:51 浏览: 265
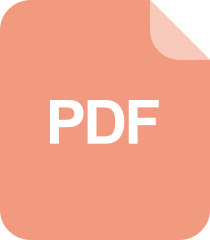
python将处理好的图像保存到指定目录下的方法
好的,可以使用 Python 中的文件操作函数将输入文本保存为 `.txt` 文件。以下是一个示例代码:
```python
text = input("请输入要保存的文本:")
save_path = input("请输入保存路径:")
# 打开文件,如果文件不存在则新建一个
with open(save_path, 'w', encoding='utf-8') as f:
# 将文本写入文件
f.write(text)
print("文件已保存到", save_path)
```
在上面的代码中,`input()` 函数用于获取用户输入的文本和保存路径。`with open()` 语句用于打开文件,`'w'` 参数表示以写入模式打开文件,如果文件不存在则会自动新建一个。`f.write(text)` 语句用于将文本写入文件。最后,打印保存成功的消息。
执行代码后,您需要在控制台中输入要保存的文本和文件路径,程序将自动保存文本到指定路径的 `.txt` 文件中。
阅读全文
相关推荐
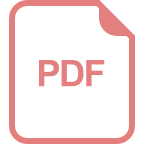
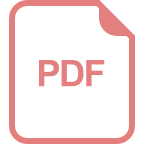















