新建一个pyqt5,要求实现点击“打开文件夹”按钮,即可自主选择所需的图片所在的文件夹,并将选中的文件夹的第一张图片展示到label中,点击“下一张”按钮,可展示下一张图片,点击“上一张”按钮,可展示上一张图片,并带有label可以展示当前图片的文件路径
时间: 2023-07-15 14:12:07 浏览: 140
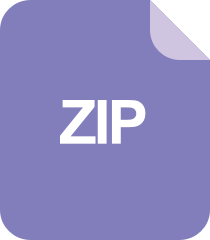
0.zip_pyqt jpg预览_pyqt5_pyqt5打开_pyqt打开文件夹_qfiledialog
好的,以下是一个简单的实现:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLabel, QHBoxLayout, QVBoxLayout, QFileDialog
from PyQt5.QtGui import QPixmap
import os
class ImageViewer(QWidget):
def __init__(self):
super().__init__()
# 初始化界面
self.initUI()
# 初始化图片列表和当前图片下标
self.image_list = []
self.current_index = -1
def initUI(self):
# 创建控件
self.label = QLabel(self)
self.path_label = QLabel(self)
self.open_button = QPushButton('打开文件夹', self)
self.prev_button = QPushButton('上一张', self)
self.next_button = QPushButton('下一张', self)
# 设置布局
h_layout = QHBoxLayout()
h_layout.addWidget(self.prev_button)
h_layout.addWidget(self.label)
h_layout.addWidget(self.next_button)
v_layout = QVBoxLayout()
v_layout.addWidget(self.path_label)
v_layout.addWidget(self.open_button)
v_layout.addLayout(h_layout)
self.setLayout(v_layout)
# 绑定按钮点击事件
self.open_button.clicked.connect(self.open_folder)
self.prev_button.clicked.connect(self.prev_image)
self.next_button.clicked.connect(self.next_image)
def open_folder(self):
# 打开文件夹选择对话框
folder_path = QFileDialog.getExistingDirectory(self, '选择文件夹')
# 获取文件夹内所有图片文件路径
image_list = [os.path.join(folder_path, file_name) for file_name in os.listdir(folder_path) if file_name.endswith(('jpg', 'png', 'jpeg'))]
if len(image_list) > 0:
# 显示第一张图片
self.current_index = 0
self.image_list = image_list
self.show_image(self.image_list[self.current_index])
def prev_image(self):
# 显示上一张图片
if self.current_index > 0:
self.current_index -= 1
self.show_image(self.image_list[self.current_index])
def next_image(self):
# 显示下一张图片
if self.current_index < len(self.image_list) - 1:
self.current_index += 1
self.show_image(self.image_list[self.current_index])
def show_image(self, image_path):
# 显示图片和路径
pixmap = QPixmap(image_path)
self.label.setPixmap(pixmap)
self.path_label.setText(image_path)
if __name__ == '__main__':
app = QApplication([])
viewer = ImageViewer()
viewer.show()
app.exec_()
```
该程序会显示一个界面,包含一个“打开文件夹”按钮、一个展示图片的label、一个展示当前图片路径的label,以及“上一张”和“下一张”按钮。当点击“打开文件夹”按钮后,会弹出文件夹选择对话框,选择所需的图片所在的文件夹后,程序会获取该文件夹内所有图片文件的路径,并显示第一张图片。点击“下一张”按钮时,程序会显示下一张图片,点击“上一张”按钮时,程序会显示上一张图片。同时,当前图片的路径也会在界面上更新。
阅读全文
相关推荐
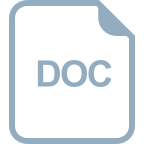
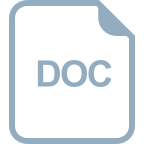
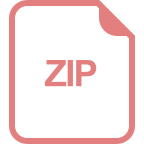
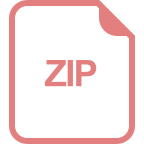
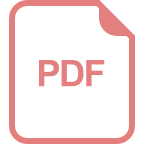
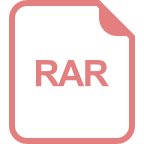
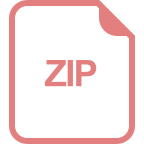
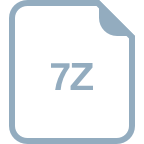
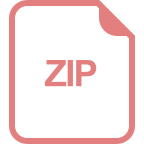
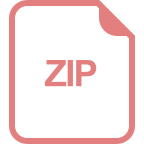
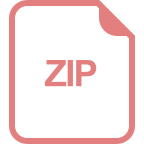
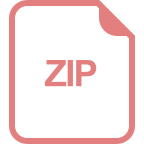
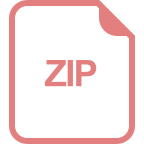
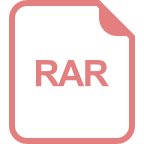
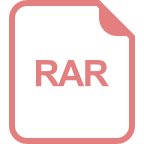
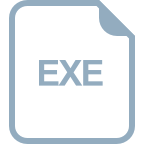
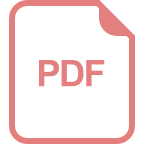
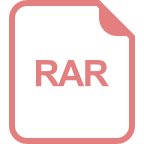