用C++写一个Ncut算法,输入为点云的ros msgs,对每一帧点云数据进行点云地面分割,点云地面以“ground_points”为ros topic发布点云话题。当前gcc版本为9.4.0
时间: 2024-12-26 20:09:17 浏览: 12
Ncut(Normalized Cuts)是一种经典的图像分割算法,它基于图论,在点云数据中通常用于将数据划分为不同的组件,比如地面上和空中的部分。在ROS (Robot Operating System) 中处理点云数据,你需要结合ROS的消息系统和C++编写算法。
首先,确保已经安装了必要的ROS库和依赖项,并理解如何使用ROS的msg(message)和srv(service)结构。下面是一个简单的步骤概述:
1. 创建`#include <ros/ros.h>` 导入ROS的基本头文件。
2. 定义一个消息类型来表示点云数据,例如`sensor_msgs::PointCloud2`。
3. 使用`ros::NodeHandle nh`来初始化ROS节点并订阅地面点云主题。
4. 实现一个函数来读取并处理`sensor_msgs::PointCloud2`的数据,计算Ncut得分。
5. 对每个帧应用Ncut算法,并从分割后的点云中提取地面部分。
6. 创建一个新的`sensor_msgs::PointCloud2`实例,包含地面点。
7. 发布`ground_points`话题,将结果传递给其他节点。
这是一个简化的示例代码片段:
```cpp
#include "sensor_msgs/PointCloud2.h"
#include "geometry_msgs/PoseStamped.h"
#include "pcl_ros/pcl_nodelet.h"
class NCutSegmentation : public pcl_ros::PCLNodelet
{
public:
void onInit() override
{
nh.subscribe("input_point_cloud", 1, &NCutSegmentation::cloudCallback, this);
pub_ground_points = nh.advertise<sensor_msgs::PointCloud2>("ground_points", 10);
}
private:
ros::Subscriber cloud_sub;
ros::Publisher pub_ground_points;
void cloudCallback(const sensor_msgs::PointCloud2ConstPtr& cloud_msg)
{
// 这里需要处理点云数据,包括转换到适合Ncut的格式
sensor_msgs::PointCloud2 ground_cloud;
// 应用Ncut算法
// ...
// 提取地面点并发布结果
// ground_cloud = extractGroundPoints(cloud_msg);
pub_ground_points.publish(ground_cloud);
}
// 你需要实现这个函数来实际执行Ncut算法和地面点提取
sensor_msgs::PointCloud2 extractGroundPoints(const sensor_msgs::PointCloud2ConstPtr& input Cloud)
{
// 略...
}
};
int main(int argc, char **argv)
{
ros::init(argc, argv, "ncut_segmentation");
NCutSegmentation node;
ros::spin();
return 0;
}
```
阅读全文
相关推荐
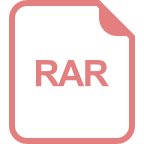
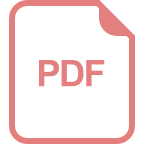

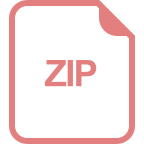
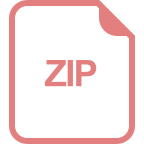
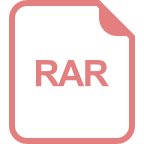
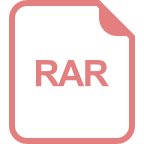
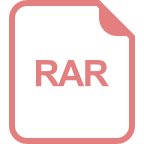
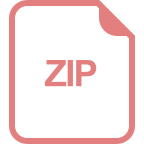
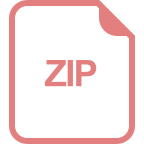
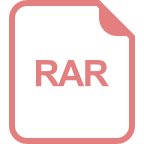
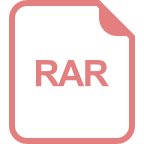
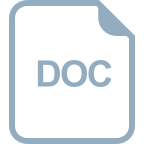
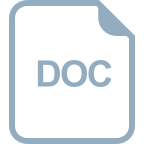
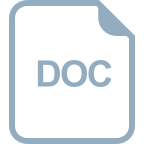
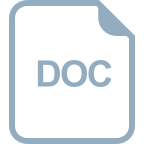
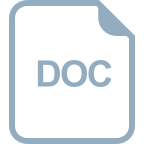
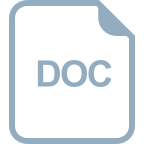