哈希表实现学生成绩管理系统
时间: 2024-06-17 18:06:36 浏览: 14
哈希表是一种非常高效的数据结构,可以用来实现学生成绩管理系统。哈希表将键映射到一个索引上,以便可以快速访问值。在学生成绩管理系统中,我们可以使用哈希表将学生的姓名映射到他们的成绩上。
具体实现方式如下:
1. 首先,定义一个哈希表数据结构,包含两个成员变量:一个数组和一个哈希函数。
2. 哈希函数接受一个字符串作为参数,返回一个整数索引。这个哈希函数可以使用标准库中的哈希函数,也可以自己编写一个简单的哈希函数。
3. 数组的每个元素都是一个链表,用于处理哈希冲突。当两个键映射到相同的索引时,它们会被添加到同一个链表中。
4. 实现成绩管理系统的基本操作,包括添加学生、删除学生、查询学生成绩等。
以下是示例代码:
```
#include <iostream>
#include <string>
#include <list>
using namespace std;
class Student {
public:
string name;
int score;
Student(string name, int score) {
this->name = name;
this->score = score;
}
};
class HashTable {
private:
static const int TABLE_SIZE = 10;
list<Student> table[TABLE_SIZE];
public:
int hashFunction(string name) {
int hash = 0;
for (int i = 0; i < name.length(); i++) {
hash += name[i];
}
return hash % TABLE_SIZE;
}
void addStudent(Student student) {
int index = hashFunction(student.name);
table[index].push_back(student);
}
void removeStudent(string name) {
int index = hashFunction(name);
for (auto it = table[index].begin(); it != table[index].end(); it++) {
if (it->name == name) {
table[index].erase(it);
break;
}
}
}
int getScore(string name) {
int index = hashFunction(name);
for (auto it = table[index].begin(); it != table[index].end(); it++) {
if (it->name == name) {
return it->score;
}
}
return -1;
}
};
int main() {
HashTable table;
table.addStudent(Student("Alice", 90));
table.addStudent(Student("Bob", 80));
table.addStudent(Student("Charlie", 70));
cout << "Alice's score is " << table.getScore("Alice") << endl;
cout << "Bob's score is " << table.getScore("Bob") << endl;
cout << "Charlie's score is " << table.getScore("Charlie") << endl;
table.removeStudent("Bob");
cout << "Bob's score is " << table.getScore("Bob") << endl;
return 0;
}
```
相关推荐
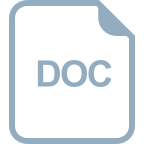
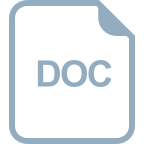














