C语言同时写几个线程(比如三个),分别写在不同的文件中,如何链接这些线程
时间: 2024-11-09 10:27:28 浏览: 6
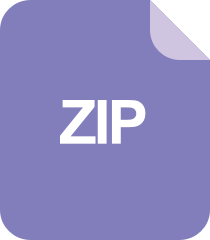
c语言文件读写txt文件操作代码示例
在C语言中,如果你有多个线程分别编写在不同的源文件中,每个线程通常都会有一个`pthread_t`类型的标识符,表示该线程。为了将这些线程链接并一起运行,你需要按照以下步骤操作:
1. **包含头文件**:首先在每个源文件(每个线程对应的.c文件)中,包括`<pthread.h>`头文件,以便使用线程相关的函数。
2. **声明线程函数**:为每个线程编写一个函数,这个函数将是`pthread_create`函数的参数。记得在函数原型前加上`void *`,因为这是线程函数的标准返回类型。
3. **定义全局变量**:在其中一个源文件(通常是主文件)中,定义一个`pthread_t`数组或者结构体数组,用于存储每个线程的标识符。这将作为线程管理的集合。
4. **创建线程**:在主函数里,对每个线程函数,调用`pthread_create`,传递线程函数、参数、堆栈大小和线程标识数组的指针。
5. **启动线程**:创建完所有线程后,你可以选择立即启动它们,或者先创建然后通过`pthread_join`等待每个线程完成。
6. **维护线程控制**:使用`pthread_join`等待所有的线程执行完毕后,再结束程序。
下面是一个简单的示例,假设你有两个线程文件:`thread1.c`和`thread2.c`,可以在主文件`main.c`中进行链接:
```c
// main.c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
typedef struct {
pthread_t thread;
} ThreadData;
ThreadData threads[2];
void* thread_func1(void* arg);
void* thread_func2(void* arg);
int main() {
// 创建线程数据并初始化
threads[0].thread = pthread_create(&threads[0].thread, NULL, thread_func1, NULL);
threads[1].thread = pthread_create(&threads[1].thread, NULL, thread_func2, NULL);
// 等待所有线程完成
for (int i = 0; i < 2; ++i) {
pthread_join(threads[i].thread, NULL);
}
return 0;
}
// thread1.c 和 thread2.c 中的线程函数定义
```
阅读全文
相关推荐
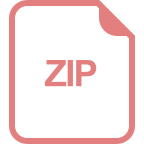
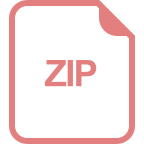
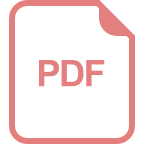
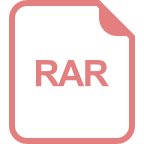
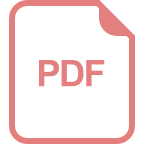
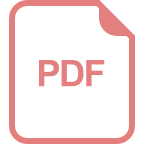
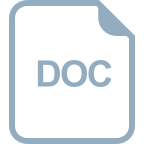
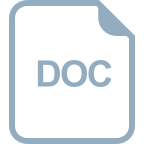
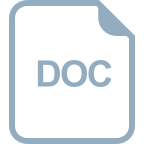
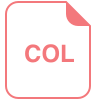
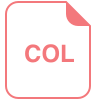
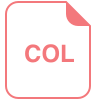
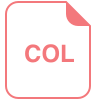
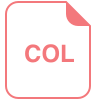
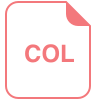
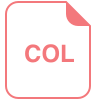
