定义一个函数对象,查找并输出所有大于给定阈值的元素,使用for_each
时间: 2024-10-13 07:06:13 浏览: 39
在C++中,你可以定义一个函数对象(functor或lambda表达式)作为`std::for_each`的遍历操作,以便找出并输出数组或容器中所有大于指定阈值的元素。下面是一个例子,使用函数对象和`std::find_if_not`配合`std::greater`来完成这一任务:
```cpp
#include <iostream>
#include <algorithm>
#include <vector>
// 函数对象,检查元素是否大于阈值
struct GreaterThanThreshold {
int threshold;
GreaterThanThreshold(int val) : threshold(val) {}
bool operator()(int elem) const {
return elem > threshold;
}
};
void print_greater_than_threshold(const std::vector<int>& nums, int threshold) {
GreaterThanThreshold greater(threshold);
for (auto& num : nums) {
if (!std::find_if_not(nums.begin(), nums.end(), greater)(num)) {
std::cout << "Element greater than or equal to threshold: " << num << '\n';
}
}
}
int main() {
std::vector<int> numbers = {5, 8, 3, 10, 6};
int threshold = 7;
print_greater_than_threshold(numbers, threshold);
return 0;
}
```
在这个例子中,`GreaterThanThreshold`函数对象接受一个阈值作为构造函数参数,`operator()`则检查每个元素是否大于该阈值。`std::find_if_not`会在容器中找到第一个不符合`greater`条件的元素(即不大于阈值的元素),如果当前元素不在结果范围内,则打印出来。
阅读全文
相关推荐
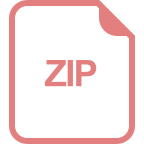
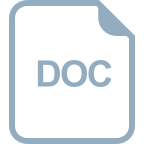
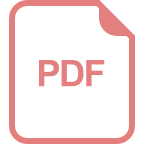

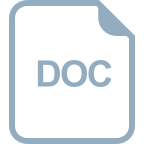
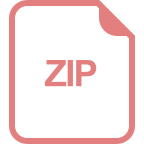
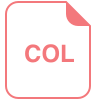
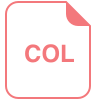
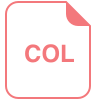
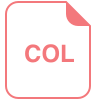
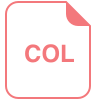
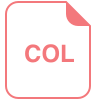
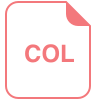
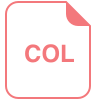
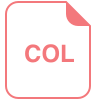
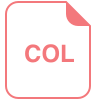
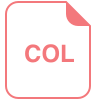
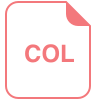
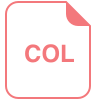
