用python写简单文字排版工具:具备删除空格、英文标点替换、英文单词大写、小写等功能。
时间: 2024-10-12 20:08:03 浏览: 51
你可以创建一个简单的Python文本处理函数,结合`re`模块(正则表达式)来实现这些功能。下面是一个简化的例子:
```python
import re
def text_formatter(text):
# 删除空格
text = text.replace(" ", "")
# 英文标点替换为下划线
punctuation_pattern = r'[!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~]'
text = re.sub(punctuation_pattern, '_', text)
# 英文单词首字母大写
words = text.split('_')
capitalized_words = [word.capitalize() for word in words]
formatted_text = ' '.join(capitalized_words)
# 全部转换为小写
formatted_text = formatted_text.lower()
return formatted_text
# 示例用法
input_text = "Hello, World! This is a Test."
formatted_output = text_formatter(input_text)
print(f"Formatted Text: {formatted_output}")
相关问题
写一个python程序,输入一个由英文字符、数字、空格和英文标点符号组成的字符串,要求将其切分为单词,去掉所有的非英文字母,然后将单词全部转换为小写,在按照字典序升序排列,每个单词用空格分隔。
可以使用正则表达式来去除非英文字母,然后使用split函数来切分字符串。最后使用sorted函数按照字典序升序排列单词。
以下是一个示例程序:
```python
import re
# 输入字符串
s = input("请输入字符串:")
# 去除非英文字母,转换为小写,切分为单词
words = re.findall(r'[a-zA-Z]+', s)
words = [word.lower() for word in words]
# 按照字典序升序排列单词
words = sorted(words)
# 输出结果
print(" ".join(words))
```
示例输入:
```
Hello World! This is a Test. 1234
```
示例输出:
```
a hello is test this world
```
在网上搜索一段英文句子,统计各单词出现的频率。 解题思路: (1)用空格替换所有标点符号 (2)用split拆分字符串,并将结果存入列表 (3)遍历列表,将单词及其出现的次数存入字典 (4)对字典排序(可按字母序,可按次数)后输出 用python写
在Python中,你可以使用`collections.Counter`类来轻松地完成这个任务。这里是一个简单的步骤说明:
首先,导入需要的模块:
```python
from collections import Counter
import re
```
然后,定义一个函数来执行上述解题思路:
```python
def word_frequency(sentence):
# 使用正则表达式替换标点符号
sentence = re.sub(r'[^\w\s]', '', sentence)
# 拆分句子成单词列表
words_list = sentence.split()
# 使用Counter计算每个单词的频率
frequency = Counter(words_list)
# 按照单词出现次数排序,返回最常出现的单词及其频率
sorted_frequency = frequency.most_common()
return sorted_frequency
# 示例使用
sentence = "Here is a sample sentence with some repeated words. Words are separated by spaces."
print(word_frequency(sentence))
```
当你运行这段代码,它会返回一个列表,其中包含按词频降序排列的元组,每个元组的第一个元素是单词,第二个元素是该单词在句子中出现的次数。
阅读全文
相关推荐
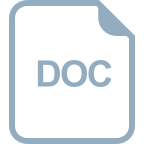
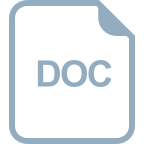
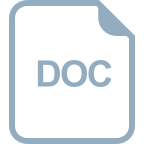
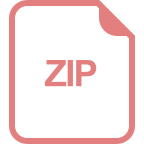












