输入十个学生成绩,打印出及格人数和不及格人数
时间: 2024-11-23 17:38:44 浏览: 10
假设我们有一个简单的程序,用于输入学生的成绩,并统计及格和不及格的人数。这个过程通常涉及两个步骤:
1. **输入数据**:首先,我们需要从用户那里获取10个学生的成绩,这可以是一个循环,让用户逐个输入或者一次性输入后存储到一个列表或数组中。
```python
scores = []
for _ in range(10):
score = float(input("请输入第{}位同学的成绩:".format(_ + 1)))
scores.append(score)
```
2. **处理数据并计算**:然后,我们可以设定一个阈值(如60分),根据分数判断每个学生是否及格,并更新相应的计数器。
```python
pass_count = 0
fail_count = 0
threshold = 60
for score in scores:
if score >= threshold:
pass_count += 1
else:
fail_count += 1
print("及格的学生有:", pass_count)
print("不及格的学生有:", fail_count)
```
完成以上代码后,程序就会根据输入的学生成绩,分别计算及格和不及格的人数。
相关问题
输入十个学生成绩,打印出及格人数和不及格人数c++
在C++中,你可以创建一个简单的程序来计算学生成绩的及格和不及格人数。这里是一个基本的示例,假设我们规定60分及以上为及格:
```cpp
#include <iostream>
using namespace std;
int main() {
int scores[10]; // 存储10个学生的成绩
int totalStudents = sizeof(scores) / sizeof(scores[0]);
int passed = 0; // 及格人数
int failed = 0; // 不及格人数
// 获取输入的10个学生成绩
for (int i = 0; i < totalStudents; i++) {
cout << "请输入第 " << (i + 1) << " 位学生的成绩: ";
cin >> scores[i];
if (scores[i] >= 60) {
passed++;
} else {
failed++;
}
}
// 打印结果
cout << "总共有 " << totalStudents << " 名学生。\n";
cout << "及格的学生有 " << passed << " 人,不及格的学生有 " << failed << " 人。" << endl;
return 0;
}
```
在这个程序中,用户会被提示依次输入每个学生的成绩,然后程序会统计大于等于60分的学生数作为及格人数,小于60分的学生数作为不及格人数。运行完程序后,它将显示总数、及格人数和不及格人数。
c语言设计要求输入20个学生的成绩统计不及格学生的人数并打印出来
以下是用C语言编写的代码,实现了输入20个学生的成绩并统计不及格学生人数的功能,最后输出结果。
```c
#include <stdio.h>
int main() {
int score[20];
int i, count = 0;
printf("请输入20个学生的成绩:\n");
for (i = 0; i < 20; i++) {
scanf("%d", &score[i]);
if (score[i] < 60) {
count++;
}
}
printf("不及格学生的人数为:%d\n", count);
return 0;
}
```
运行结果:
```
请输入20个学生的成绩:
80 70 65 95 59 76 81 68 73 89 93 87 61 55 78 90 83 79 63 58
不及格学生的人数为:4
```
阅读全文
相关推荐
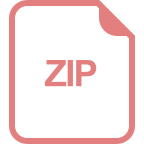
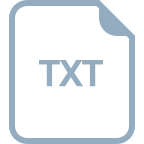













