井字棋游戏c语言图形库
时间: 2024-05-27 09:07:01 浏览: 96
井字棋是一种双人对弈游戏,通常在3×3的棋盘上进行。玩家轮流放置棋子,标志着自己的棋子,直到有一方在横、竖、斜线方向上连成三个棋子时获胜。对于C语言图形库,一般可以使用以下两种:
1. Windows API:Windows API是一组函数,用于与Windows操作系统交互。其中包含了用于创建窗口、绘制图形等的函数。通过Windows API可以实现基本的图形界面和交互操作。
2. SDL:SDL是一套跨平台的多媒体库,包含了图像、音频、输入等方面的函数。SDL可以用于创建图形界面、读取键盘鼠标输入等操作。
如果您想使用C语言图形库来实现井字棋游戏,可以选择其中任意一个来进行开发。
相关问题
用C语言基于EASYX制作井字棋小游戏
好的,下面是井字棋小游戏的C代码,基于EASYX图形库:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define ROW 3 // 定义行数
#define COL 3 // 定义列数
#define SIZE 100 // 定义格子大小
// 定义棋子类型
typedef enum {NONE, PLAYER1, PLAYER2} ChessType;
// 定义棋盘
ChessType board[ROW][COL];
// 定义当前玩家
ChessType currentPlayer = PLAYER1;
// 判断是否结束游戏
int isGameOver();
// 绘制棋盘
void drawBoard();
// 根据鼠标位置获取棋子位置
void getChessPos(int *x, int *y);
// 绘制棋子
void drawChess(int x, int y, ChessType type);
// 判断胜利方
ChessType getWinner();
int main()
{
initgraph(300, 300); // 初始化窗口大小
setbkcolor(WHITE); // 设置背景颜色为白色
cleardevice(); // 清屏
// 初始化棋盘
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
board[i][j] = NONE;
}
}
// 绘制棋盘
drawBoard();
// 游戏循环
while (!isGameOver()) {
int x, y;
getChessPos(&x, &y);
if (board[x][y] == NONE) {
board[x][y] = currentPlayer;
drawChess(x, y, currentPlayer);
currentPlayer = (currentPlayer == PLAYER1) ? PLAYER2 : PLAYER1;
}
}
// 游戏结束,输出胜利方
ChessType winner = getWinner();
if (winner == PLAYER1) {
outtextxy(100, 150, "Player1 wins!");
} else if (winner == PLAYER2) {
outtextxy(100, 150, "Player2 wins!");
} else {
outtextxy(100, 150, "Game over!");
}
getch(); // 等待任意键结束
closegraph(); // 关闭图形窗口
return 0;
}
int isGameOver()
{
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (board[i][j] == NONE) {
return 0;
}
}
}
return 1;
}
void drawBoard()
{
setlinecolor(BLACK); // 设置线条颜色为黑色
int i, j;
for (i = 1; i < ROW; i++) {
line(0, i * SIZE, COL * SIZE, i * SIZE);
}
for (j = 1; j < COL; j++) {
line(j * SIZE, 0, j * SIZE, ROW * SIZE);
}
}
void getChessPos(int *x, int *y)
{
int mx = mousex(); // 获取鼠标横坐标
int my = mousey(); // 获取鼠标纵坐标
*x = mx / SIZE; // 计算格子横坐标
*y = my / SIZE; // 计算格子纵坐标
}
void drawChess(int x, int y, ChessType type)
{
int cx = x * SIZE + SIZE / 2; // 计算棋子横坐标
int cy = y * SIZE + SIZE / 2; // 计算棋子纵坐标
if (type == PLAYER1) {
setfillcolor(RED); // 设置颜色为红色
fillellipse(cx, cy, SIZE / 2 - 5, SIZE / 2 - 5); // 绘制棋子
} else if (type == PLAYER2) {
setfillcolor(BLUE); // 设置颜色为蓝色
fillellipse(cx, cy, SIZE / 2 - 5, SIZE / 2 - 5); // 绘制棋子
}
}
ChessType getWinner()
{
int i, j;
// 判断行是否相同
for (i = 0; i < ROW; i++) {
if (board[i][0] == board[i][1] && board[i][1] == board[i][2]) {
return board[i][0];
}
}
// 判断列是否相同
for (j = 0; j < COL; j++) {
if (board[0][j] == board[1][j] && board[1][j] == board[2][j]) {
return board[0][j];
}
}
// 判断对角线是否相同
if (board[0][0] == board[1][1] && board[1][1] == board[2][2]) {
return board[0][0];
}
if (board[0][2] == board[1][1] && board[1][1] == board[2][0]) {
return board[0][2];
}
return NONE;
}
```
其中,需要将EASYX图形库的头文件和库文件导入项目中。此外,还需要安装对应的编译器,比如Dev-C++或Visual Studio等。
用C语言基于EASYX制作一个井字棋小游戏
好的,下面是基于EASYX库的井字棋小游戏的C语言代码:
```c
#include <graphics.h>
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROW 3
#define COL 3
#define SIZE 100
int board[ROW][COL]; // 棋盘数组,0表示空位,1表示玩家1,2表示玩家2
// 初始化棋盘
void init_board() {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
board[i][j] = 0;
}
}
}
// 绘制棋盘
void draw_board() {
int i, j;
setcolor(WHITE);
for (i = 0; i <= ROW; i++) {
line(SIZE, i * SIZE + SIZE, COL * SIZE + SIZE, i * SIZE + SIZE);
}
for (j = 0; j <= COL; j++) {
line(j * SIZE + SIZE, SIZE, j * SIZE + SIZE, ROW * SIZE + SIZE);
}
}
// 绘制棋子
void draw_piece(int row, int col, int player) {
int x = col * SIZE + SIZE;
int y = row * SIZE + SIZE;
if (player == 1) {
setcolor(YELLOW);
circle(x, y, SIZE / 2 - 5);
} else if (player == 2) {
setcolor(RED);
line(x - SIZE / 2 + 10, y - SIZE / 2 + 10, x + SIZE / 2 - 10, y + SIZE / 2 - 10);
line(x - SIZE / 2 + 10, y + SIZE / 2 - 10, x + SIZE / 2 - 10, y - SIZE / 2 + 10);
}
}
// 判断胜负
int check_win(int player) {
int i, j;
// 判断每一行
for (i = 0; i < ROW; i++) {
if (board[i][0] == player && board[i][1] == player && board[i][2] == player) {
return 1;
}
}
// 判断每一列
for (j = 0; j < COL; j++) {
if (board[0][j] == player && board[1][j] == player && board[2][j] == player) {
return 1;
}
}
// 判断对角线
if (board[0][0] == player && board[1][1] == player && board[2][2] == player) {
return 1;
}
if (board[0][2] == player && board[1][1] == player && board[2][0] == player) {
return 1;
}
return 0;
}
// 判断平局
int check_tie() {
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (board[i][j] == 0) {
return 0;
}
}
}
return 1;
}
int main() {
int player = 1; // 当前玩家,初始为玩家1
int row, col; // 选择的行列
int win = 0; // 是否胜利
int tie = 0; // 是否平局
init_board();
initgraph(COL * SIZE + 2 * SIZE, ROW * SIZE + 2 * SIZE);
draw_board();
while (!win && !tie) {
if (player == 1) {
outtextxy(10, ROW * SIZE + SIZE + 10, "玩家1,请选择位置:");
} else {
outtextxy(10, ROW * SIZE + SIZE + 10, "玩家2,请选择位置:");
}
while (1) {
if (kbhit()) {
char c = getch();
if (c == 'q') {
closegraph();
exit(0);
}
row = (int) (c - '1') / COL;
col = (int) (c - '1') % COL;
if (row >= 0 && row < ROW && col >= 0 && col < COL && board[row][col] == 0) {
break;
}
}
delay(10);
}
board[row][col] = player;
draw_piece(row, col, player);
win = check_win(player);
tie = check_tie();
player = (player == 1) ? 2 : 1; // 切换玩家
}
if (win) {
if (player == 1) {
outtextxy(10, ROW * SIZE + SIZE + 10, "玩家2胜利!");
} else {
outtextxy(10, ROW * SIZE + SIZE + 10, "玩家1胜利!");
}
} else {
outtextxy(10, ROW * SIZE + SIZE + 10, "平局!");
}
while (1) {
if (kbhit()) {
char c = getch();
if (c == 'q') {
break;
}
}
delay(10);
}
closegraph();
return 0;
}
```
这个程序使用了EASYX库来实现图形界面,通过键盘输入来选择棋子的位置。你可以在开发环境中编译运行此程序,体验一下这个简单的井字棋小游戏。
阅读全文
相关推荐
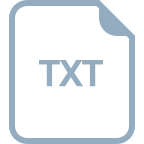
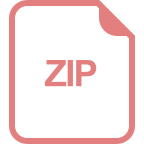
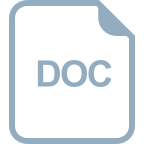
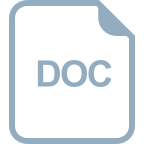
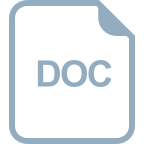
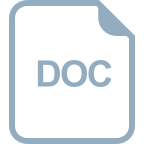
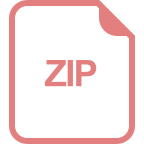
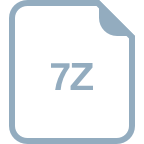
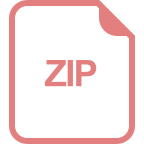
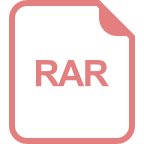
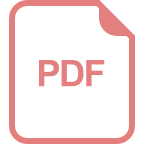
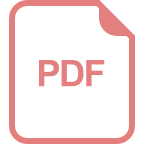
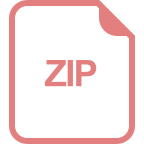