java批量解压文件
时间: 2023-10-14 22:56:59 浏览: 170
您可以使用Java的ZipInputStream类来实现批量解压文件。以下是一个简单的示例代码:
```java
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class UnzipFiles {
public static void main(String[] args) {
String zipFilePath = "path/to/your/zipfile.zip";
String destDir = "path/to/destination/folder";
try {
unzip(zipFilePath, destDir);
System.out.println("文件解压成功!");
} catch (IOException e) {
System.out.println("文件解压失败:" + e.getMessage());
}
}
public static void unzip(String zipFilePath, String destDir) throws IOException {
File dir = new File(destDir);
// 创建目标文件夹
if (!dir.exists()) {
dir.mkdirs();
}
FileInputStream fis = new FileInputStream(zipFilePath);
ZipInputStream zipIn = new ZipInputStream(fis);
ZipEntry entry = zipIn.getNextEntry();
while (entry != null) {
String filePath = destDir + File.separator + entry.getName();
if (!entry.isDirectory()) {
// 如果entry是文件,则创建对应的文件
extractFile(zipIn, filePath);
} else {
// 如果entry是文件夹,则创建对应的文件夹
File dirToCreate = new File(filePath);
dirToCreate.mkdirs();
}
zipIn.closeEntry();
entry = zipIn.getNextEntry();
}
zipIn.close();
fis.close();
}
private static void extractFile(ZipInputStream zipIn, String filePath) throws IOException {
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(filePath));
byte[] buffer = new byte[1024];
int read;
while ((read = zipIn.read(buffer)) != -1) {
bos.write(buffer, 0, read);
}
bos.close();
}
}
```
您只需要将`zipFilePath`和`destDir`变量替换为您自己的文件路径和目标文件夹路径,然后运行这段代码,它将会解压指定的ZIP文件到目标文件夹中。请确保您已经正确引入Java的IO相关类和zip包。
阅读全文
相关推荐

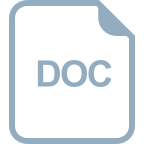
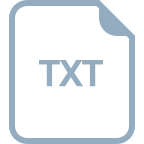
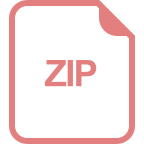
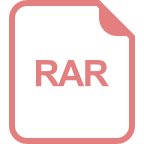
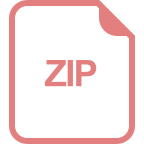
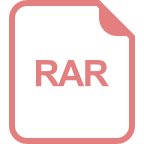
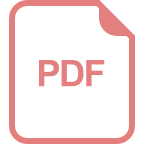
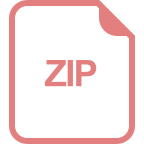
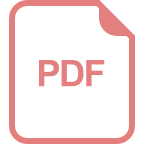
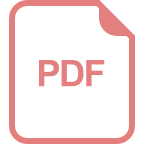
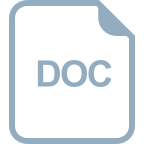
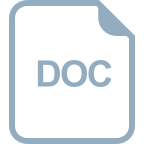


