WPF MVVM根据是否选择某个按钮切换List BOX绑定的数据源,数据源是两个自定义类型不同的ObservableCollection集合,用代码演示
时间: 2024-03-02 20:53:41 浏览: 21
好的,以下是一个简单的WPF MVVM示例,根据是否选择某个按钮切换List Box绑定的数据源,数据源是两个自定义类型不同的ObservableCollection集合:
首先,我们需要定义两个自定义类型的类,例如:
```csharp
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Animal
{
public string Name { get; set; }
public string Type { get; set; }
}
```
然后,我们需要在ViewModel中定义两个ObservableCollection属性来保存这两个类型的数据,例如:
```csharp
public class MainViewModel : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public ObservableCollection<Person> Persons { get; set; }
public ObservableCollection<Animal> Animals { get; set; }
private bool _isPersonSelected;
public bool IsPersonSelected
{
get { return _isPersonSelected; }
set
{
if (_isPersonSelected != value)
{
_isPersonSelected = value;
OnPropertyChanged(nameof(IsPersonSelected));
OnPropertyChanged(nameof(Data));
}
}
}
public IEnumerable<object> Data
{
get { return IsPersonSelected ? Persons.Cast<object>() : Animals.Cast<object>(); }
}
private void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
```
在这个ViewModel中,我们定义了两个ObservableCollection属性来保存Person和Animal类型的数据。另外,我们定义了一个IsPersonSelected属性来表示用户是否选择了Person类型的数据。当IsPersonSelected属性发生变化时,我们通过调用OnPropertyChanged方法来通知View更新Data属性。Data属性是一个只读属性,它根据IsPersonSelected属性返回Persons或Animals集合的内容。
最后,我们需要在View中绑定这些属性,例如:
```xaml
<Window x:Class="WpfApp1.MainWindow"
...
xmlns:local="clr-namespace:WpfApp1"
Title="MainWindow" Height="450" Width="800">
<Window.DataContext>
<local:MainViewModel />
</Window.DataContext>
<Grid>
<StackPanel Orientation="Horizontal" Margin="10">
<RadioButton Content="Person" IsChecked="{Binding IsPersonSelected, Mode=TwoWay}" Margin="5" />
<RadioButton Content="Animal" IsChecked="{Binding IsPersonSelected, Converter={StaticResource InverseBooleanConverter}, Mode=TwoWay}" Margin="5" />
</StackPanel>
<ListBox ItemsSource="{Binding Data}" Margin="10">
<ListBox.ItemTemplate>
<DataTemplate DataType="{x:Type local:Person}">
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" Margin="5" />
<TextBlock Text="{Binding Age}" Margin="5" />
</StackPanel>
</DataTemplate>
<DataTemplate DataType="{x:Type local:Animal}">
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding Name}" Margin="5" />
<TextBlock Text="{Binding Type}" Margin="5" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Window>
```
在这个View中,我们首先设置了DataContext为MainViewModel的实例。然后,我们使用两个RadioButton控件来让用户选择Person或Animal类型的数据。这些RadioButton控件的IsChecked属性都绑定到MainViewModel的IsPersonSelected属性。注意,第二个RadioButton控件使用了一个Boolean转换器来反转IsPersonSelected属性的值。
接下来,我们使用一个ListBox控件来显示数据。这个ListBox控件的ItemsSource属性绑定到MainViewModel的Data属性。由于Data属性返回的是一个IEnumerable<object>类型,所以我们需要为每个数据类型定义一个DataTemplate。在这些DataTemplate中,我们使用TextBlock控件来显示Person或Animal类型的数据的属性。
当用户选择了不同的RadioButton控件时,ListBox控件的数据源会自动切换到不同的ObservableCollection集合,并且显示不同的数据类型。
相关推荐
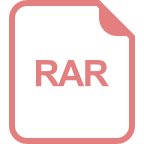














