可以使用findContours函数得到contours 而contours可以作为 cv2.filter2D函数的输入嘛
时间: 2024-05-25 22:10:46 浏览: 76
不可以,`cv2.findContours()` 函数返回的是轮廓列表,每个轮廓都是一组点的集合,不能作为 `cv2.filter2D()` 函数的输入。`cv2.filter2D()` 函数需要输入一个图像矩阵,用于进行卷积操作。如果你想要在轮廓上进行卷积操作,可以考虑先将轮廓转换为二值图像,然后再进行卷积操作。你可以使用 `cv2.drawContours()` 函数将轮廓绘制到一个空白的图像上,得到一个二值图像,然后再将该图像输入到 `cv2.filter2D()` 函数中进行卷积操作。
相关问题
初学者如何使用OpenCV实现基本的图像处理功能?请结合《唐老师带你轻松入门OpenCV计算机视觉》进行说明。
对于初学者来说,掌握OpenCV实现基本图像处理功能是打开计算机视觉世界大门的第一步。《唐老师带你轻松入门OpenCV计算机视觉》这份资源提供了一个从零开始的学习途径,非常适合对图像处理感兴趣的初学者。要使用OpenCV实现基本图像处理,首先需要了解一些关键概念和操作步骤:
参考资源链接:[唐老师带你轻松入门OpenCV计算机视觉](https://wenku.csdn.net/doc/129mr775h0?spm=1055.2569.3001.10343)
1. 环境搭建:安装OpenCV库,确保可以在自己的编程环境中使用。对于Python用户,通常通过pip安装cv2库:`pip install opencv-python`。
2. 图像读取与显示:使用cv2.imread()函数读取图片,cv2.imshow()显示图片。例如:
```python
import cv2
img = cv2.imread('image.jpg') # 读取图片
cv2.imshow('image', img) # 显示图片
cv2.waitKey(0) # 等待任意键退出
cv2.destroyAllWindows() # 关闭所有窗口
```
3. 图像转换:包括颜色空间转换,如将彩色图像转换为灰度图,使用cv2.cvtColor()函数实现。
```python
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # 彩色转灰度
```
4. 图像滤波:使用cv2.filter2D()或内置的滤波函数如cv2.GaussianBlur(),cv2.blur()等对图像进行平滑处理。
```python
blurred_img = cv2.GaussianBlur(gray_img, (5, 5), 0) # 高斯模糊
```
5. 边缘检测:利用Canny算法进行边缘检测,使用cv2.Canny()函数。
```python
edges = cv2.Canny(blurred_img, 100, 200) # 边缘检测
```
6. 图像形态学操作:包括膨胀、腐蚀、开运算和闭运算等,这些可以帮助我们进行图像的形态学变换。
```python
kernel = np.ones((5, 5), np.uint8)
dilated_img = cv2.dilate(edges, kernel, iterations=1) # 膨胀操作
```
7. 对象检测与分析:可以使用轮廓查找、对象属性获取等技术进行对象的检测和分析。
```python
contours, hierarchy = cv2.findContours(dilated_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
# 可以对轮廓进行进一步处理
```
以上步骤涵盖了初学者使用OpenCV进行基本图像处理的几个关键步骤。通过阅读《唐老师带你轻松入门OpenCV计算机视觉》,初学者不仅能够了解到这些基本操作的细节,还可以通过实例加深理解并进行实际操作练习。这对于理解和掌握OpenCV图像处理功能的实现非常重要,也为后续更高级的计算机视觉项目打下坚实的基础。
参考资源链接:[唐老师带你轻松入门OpenCV计算机视觉](https://wenku.csdn.net/doc/129mr775h0?spm=1055.2569.3001.10343)
如何用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,需要分析大量图像以确保结果的真实性,超声图像的文件路径是‘D:\zzz\zus2’,自然图像的文件路径是‘D:\zzz\zna2’,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细复杂代码,不使用greycomatrix, greycoprops
这是一个复杂的图像处理问题,需要使用多种图像处理技术和机器学习方法。以下是一个简单的代码示例,用于说明如何从大量超声图像和自然图像中提取特征,并使用概率分布进行差异性分析。
首先,我们需要导入必要的库:
```python
import os
import cv2
import numpy as np
from sklearn.utils import shuffle
from sklearn.model_selection import train_test_split
from matplotlib import pyplot as plt
```
接下来,我们需要定义一些函数来提取图像的不同特征。在这个示例中,我们将使用颜色直方图、Gabor滤波器和形状描述符。
```python
def color_histogram(image, mask=None, bins=(8, 8, 8)):
hist = cv2.calcHist([image], [0, 1, 2], mask, bins,
[0, 256, 0, 256, 0, 256])
hist = cv2.normalize(hist, hist)
return hist.flatten()
def gabor_filter(image, ksize=(31, 31), sigma=5.0, theta=np.pi/4, lambd=10.0, gamma=0.5):
kernel = cv2.getGaborKernel(ksize, sigma, theta, lambd, gamma)
filtered = cv2.filter2D(image, cv2.CV_8UC3, kernel)
return filtered.flatten()
def shape_descriptor(image):
contours, _ = cv2.findContours(image, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contour = max(contours, key=cv2.contourArea)
moments = cv2.moments(contour)
hu_moments = cv2.HuMoments(moments)
return hu_moments.flatten()
```
接下来,我们需要定义一个函数来加载图像并提取所需的特征。
```python
def load_images(path, feature_extractor):
images = []
labels = []
for label, directory in enumerate(os.listdir(path)):
subdirectory = os.path.join(path, directory)
for filename in os.listdir(subdirectory):
filepath = os.path.join(subdirectory, filename)
image = cv2.imread(filepath)
feature = feature_extractor(image)
images.append(feature)
labels.append(label)
return np.array(images), np.array(labels)
```
现在我们可以使用这些函数来加载图像并提取所需的特征。
```python
# Load ultrasound images
ultrasound_path = 'D:\\zzz\\zus2'
ultrasound_images, ultrasound_labels = load_images(ultrasound_path, lambda x: np.concatenate((
color_histogram(x),
gabor_filter(x),
shape_descriptor(cv2.cvtColor(x, cv2.COLOR_BGR2GRAY))
)))
# Load natural images
natural_path = 'D:\\zzz\\zna2'
natural_images, natural_labels = load_images(natural_path, lambda x: np.concatenate((
color_histogram(x),
gabor_filter(x),
shape_descriptor(cv2.cvtColor(x, cv2.COLOR_BGR2GRAY))
)))
```
现在我们可以将数据集拆分为训练集和测试集,并训练一个简单的分类器来区分超声图像和自然图像。
```python
# Combine ultrasound and natural images
images = np.concatenate((ultrasound_images, natural_images))
labels = np.concatenate((ultrasound_labels, natural_labels))
# Shuffle data
images, labels = shuffle(images, labels)
# Split data into train and test sets
x_train, x_test, y_train, y_test = train_test_split(images, labels, test_size=0.2)
# Train a classifier (e.g. SVM)
from sklearn.svm import SVC
classifier = SVC(kernel='linear')
classifier.fit(x_train, y_train)
# Evaluate classifier on test set
accuracy = classifier.score(x_test, y_test)
print('Accuracy:', accuracy)
```
最后,我们可以使用概率分布来比较超声图像和自然图像的特征差异。
```python
# Compute probability distributions for ultrasound and natural images
ultrasound_prob = np.mean(classifier.predict_proba(ultrasound_images), axis=0)
natural_prob = np.mean(classifier.predict_proba(natural_images), axis=0)
# Plot probability distributions
plt.bar(range(2), ultrasound_prob, color='blue', label='Ultrasound')
plt.bar(range(2), natural_prob, color='green', bottom=ultrasound_prob, label='Natural')
plt.xticks(range(2), ('Ultrasound', 'Natural'))
plt.xlabel('Image Type')
plt.ylabel('Probability')
plt.legend()
plt.show()
```
这些代码片段只是一个示例,您可能需要根据您的数据和实际情况进行修改。但是,这应该足以为您提供一个基本的框架来处理这个问题。
阅读全文
相关推荐
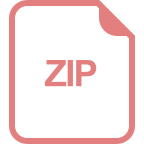
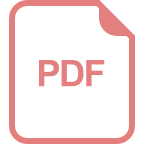
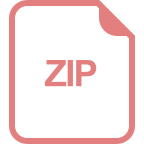
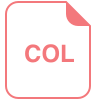
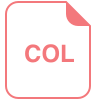
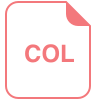
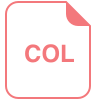
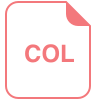
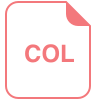
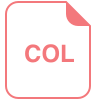
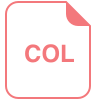
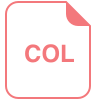
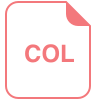
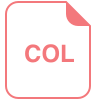
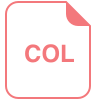
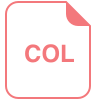