基于matlab的gui简易计算器完成科学函数的功能
时间: 2023-12-14 17:03:04 浏览: 246
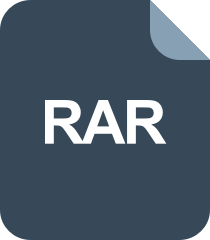
基于MATLAB GUI 计算器实现
好的,我可以为您提供一些思路。首先,您需要创建一个MATLAB GUI界面,该界面包括数字按钮、运算符按钮、科学函数按钮等。然后,您需要编写MATLAB代码来处理这些按钮的点击事件,并将结果显示在GUI界面上。
对于科学函数的功能,您需要在MATLAB代码中编写相应的函数来处理这些计算。例如,您可以编写一个处理正弦函数的函数,一个处理余弦函数的函数等等。
以下是一个简单的MATLAB GUI计算器的示例代码,其中包括一些基本的数学和科学函数的计算:
```matlab
function simple_calculator
% Create a simple calculator GUI
% Create the GUI figure
hFig = figure('Name', 'Simple Calculator', 'NumberTitle', 'off', ...
'MenuBar', 'none', 'ToolBar', 'none', 'Position', [100 100 300 400]);
% Create the GUI components
hOutput = uicontrol('Style', 'edit', 'Position', [30 350 240 40], ...
'HorizontalAlignment', 'right', 'FontSize', 16);
hButton1 = uicontrol('Style', 'pushbutton', 'String', '1', ...
'Position', [30 290 50 50], 'FontSize', 16, 'Callback', @button1_Callback);
hButton2 = uicontrol('Style', 'pushbutton', 'String', '2', ...
'Position', [90 290 50 50], 'FontSize', 16, 'Callback', @button2_Callback);
hButton3 = uicontrol('Style', 'pushbutton', 'String', '3', ...
'Position', [150 290 50 50], 'FontSize', 16, 'Callback', @button3_Callback);
hButton4 = uicontrol('Style', 'pushbutton', 'String', '4', ...
'Position', [30 230 50 50], 'FontSize', 16, 'Callback', @button4_Callback);
hButton5 = uicontrol('Style', 'pushbutton', 'String', '5', ...
'Position', [90 230 50 50], 'FontSize', 16, 'Callback', @button5_Callback);
hButton6 = uicontrol('Style', 'pushbutton', 'String', '6', ...
'Position', [150 230 50 50], 'FontSize', 16, 'Callback', @button6_Callback);
hButton7 = uicontrol('Style', 'pushbutton', 'String', '7', ...
'Position', [30 170 50 50], 'FontSize', 16, 'Callback', @button7_Callback);
hButton8 = uicontrol('Style', 'pushbutton', 'String', '8', ...
'Position', [90 170 50 50], 'FontSize', 16, 'Callback', @button8_Callback);
hButton9 = uicontrol('Style', 'pushbutton', 'String', '9', ...
'Position', [150 170 50 50], 'FontSize', 16, 'Callback', @button9_Callback);
hButton0 = uicontrol('Style', 'pushbutton', 'String', '0', ...
'Position', [90 110 50 50], 'FontSize', 16, 'Callback', @button0_Callback);
hButtonPlus = uicontrol('Style', 'pushbutton', 'String', '+', ...
'Position', [210 290 50 50], 'FontSize', 16, 'Callback', @buttonPlus_Callback);
hButtonMinus = uicontrol('Style', 'pushbutton', 'String', '-', ...
'Position', [210 230 50 50], 'FontSize', 16, 'Callback', @buttonMinus_Callback);
hButtonTimes = uicontrol('Style', 'pushbutton', 'String', '*', ...
'Position', [210 170 50 50], 'FontSize', 16, 'Callback', @buttonTimes_Callback);
hButtonDivide = uicontrol('Style', 'pushbutton', 'String', '/', ...
'Position', [210 110 50 50], 'FontSize', 16, 'Callback', @buttonDivide_Callback);
hButtonEquals = uicontrol('Style', 'pushbutton', 'String', '=', ...
'Position', [150 110 50 50], 'FontSize', 16, 'Callback', @buttonEquals_Callback);
hButtonClear = uicontrol('Style', 'pushbutton', 'String', 'C', ...
'Position', [30 110 50 50], 'FontSize', 16, 'Callback', @buttonClear_Callback);
hButtonSin = uicontrol('Style', 'pushbutton', 'String', 'sin', ...
'Position', [30 50 50 50], 'FontSize', 16, 'Callback', @buttonSin_Callback);
hButtonCos = uicontrol('Style', 'pushbutton', 'String', 'cos', ...
'Position', [90 50 50 50], 'FontSize', 16, 'Callback', @buttonCos_Callback);
hButtonTan = uicontrol('Style', 'pushbutton', 'String', 'tan', ...
'Position', [150 50 50 50], 'FontSize', 16, 'Callback', @buttonTan_Callback);
% Initialize the calculator variables
num1 = '';
num2 = '';
operator = '';
result = '';
% Define the button callback functions
function button1_Callback(hObject,eventdata)
numInput('1');
end
function button2_Callback(hObject,eventdata)
numInput('2');
end
function button3_Callback(hObject,eventdata)
numInput('3');
end
function button4_Callback(hObject,eventdata)
numInput('4');
end
function button5_Callback(hObject,eventdata)
numInput('5');
end
function button6_Callback(hObject,eventdata)
numInput('6');
end
function button7_Callback(hObject,eventdata)
numInput('7');
end
function button8_Callback(hObject,eventdata)
numInput('8');
end
function button9_Callback(hObject,eventdata)
numInput('9');
end
function button0_Callback(hObject,eventdata)
numInput('0');
end
function buttonPlus_Callback(hObject,eventdata)
operatorInput('+');
end
function buttonMinus_Callback(hObject,eventdata)
operatorInput('-');
end
function buttonTimes_Callback(hObject,eventdata)
operatorInput('*');
end
function buttonDivide_Callback(hObject,eventdata)
operatorInput('/');
end
function buttonEquals_Callback(hObject,eventdata)
equalsInput();
end
function buttonClear_Callback(hObject,eventdata)
clearInput();
end
function buttonSin_Callback(hObject,eventdata)
sinInput();
end
function buttonCos_Callback(hObject,eventdata)
cosInput();
end
function buttonTan_Callback(hObject,eventdata)
tanInput();
end
% Define the input functions
function numInput(num)
if isempty(operator)
num1 = [num1 num];
set(hOutput, 'String', num1);
else
num2 = [num2 num];
set(hOutput, 'String', num2);
end
end
function operatorInput(op)
operator = op;
set(hOutput, 'String', operator);
end
function equalsInput()
if ~isempty(num1) && ~isempty(num2) && ~isempty(operator)
num1 = str2num(num1);
num2 = str2num(num2);
switch operator
case '+'
result = num1 + num2;
case '-'
result = num1 - num2;
case '*'
result = num1 * num2;
case '/'
result = num1 / num2;
end
set(hOutput, 'String', num2str(result));
num1 = num2str(result);
num2 = '';
operator = '';
end
end
function clearInput()
num1 = '';
num2 = '';
operator = '';
result = '';
set(hOutput, 'String', '');
end
function sinInput()
if ~isempty(num1)
num1 = str2num(num1);
result = sin(num1);
set(hOutput, 'String', num2str(result));
num1 = num2str(result);
num2 = '';
operator = '';
end
end
function cosInput()
if ~isempty(num1)
num1 = str2num(num1);
result = cos(num1);
set(hOutput, 'String', num2str(result));
num1 = num2str(result);
num2 = '';
operator = '';
end
end
function tanInput()
if ~isempty(num1)
num1 = str2num(num1);
result = tan(num1);
set(hOutput, 'String', num2str(result));
num1 = num2str(result);
num2 = '';
operator = '';
end
end
end
```
希望这可以帮助您开始开发您自己的MATLAB GUI计算器,如果您有任何问题,请随时问我。
阅读全文
相关推荐
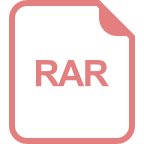
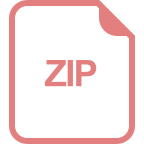
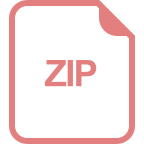
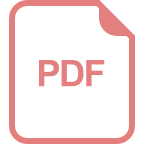
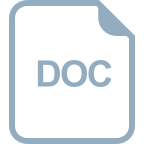
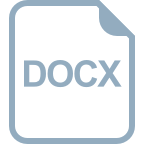
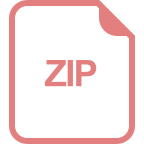
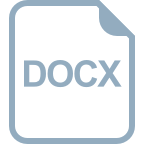
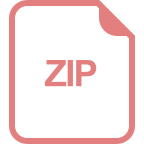
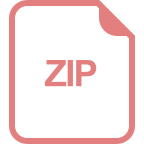
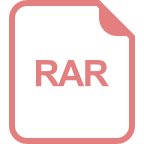
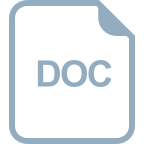
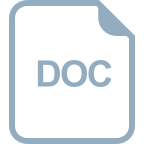

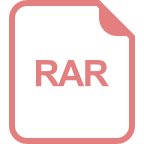