使用c语言编写函数,将两个字符串s和t连接起来。s、t的长度均不超过100。不得使用系统库函数。 若s为FirstString--,t为SecondString,则输出结果为FirstString--SecondString。
时间: 2024-02-24 14:55:01 浏览: 66
可以使用指针和循环来实现字符串连接的功能,可以按照以下方法来编写函数:
```c
void string_concat(char *s, char *t) {
// 找到s的末尾
while (*s != '\0') {
s++;
}
// 将t复制到s后面
while (*t != '\0') {
*s = *t;
s++;
t++;
}
// 添加字符串结束标志
*s = '\0';
}
```
在上述代码中,第一个while循环用于找到字符串s的末尾,第二个while循环用于将字符串t复制到s的末尾,最后添加字符串结束标志'\0'。可以在main函数中调用该函数实现字符串连接的功能:
```c
#include <stdio.h>
void string_concat(char *s, char *t);
int main() {
char s[101] = "FirstString--";
char t[101] = "SecondString";
string_concat(s, t);
printf("%s\n", s); // 输出结果为FirstString--SecondString
return 0;
}
void string_concat(char *s, char *t) {
// 找到s的末尾
while (*s != '\0') {
s++;
}
// 将t复制到s后面
while (*t != '\0') {
*s = *t;
s++;
t++;
}
// 添加字符串结束标志
*s = '\0';
}
```
注意,为了确保字符串s有足够的空间来存储连接后的字符串,需要保证字符串s的长度不小于两个字符串长度之和。
阅读全文
相关推荐
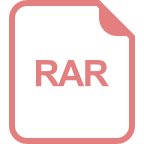
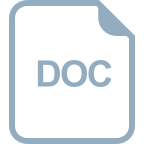
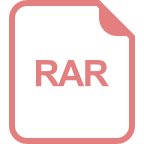
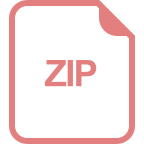
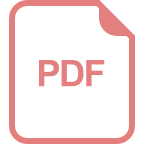
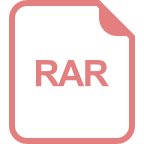
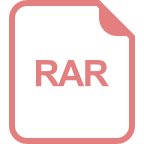
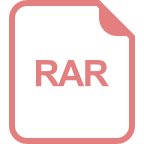
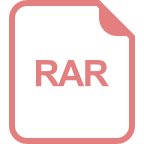
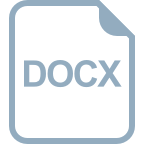
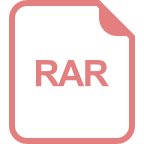
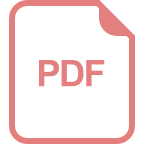
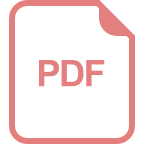
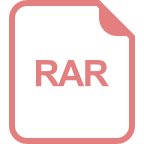