java在子类中显性调用父类属性
时间: 2023-10-04 10:10:47 浏览: 150
在Java中,可以使用`super`关键字来显式地调用父类属性。例如,如果父类有一个名为`name`的属性,而子类想要访问该属性,可以使用`super.name`来访问它。以下是一个示例:
```java
class Animal {
String name = "Animal";
}
class Dog extends Animal {
String name = "Dog";
void printNames() {
System.out.println("Name of the dog: " + name);
System.out.println("Name of the animal: " + super.name);
}
}
public class Main {
public static void main(String[] args) {
Dog dog = new Dog();
dog.printNames();
}
}
```
在上面的示例中,`Dog`类继承自`Animal`类,并且它们都有一个名为`name`的属性。在`printNames()`方法中,我们使用`super.name`来显式地访问父类的`name`属性,以便我们可以同时访问子类和父类的属性。
相关问题
java把子类属性赋值给父类属性
在Java中,您可以通过使用super关键字来访问父类的属性和方法。如果您想在子类中将子类的属性值赋给父类的属性,可以在子类的构造函数中使用super关键字来调用父类的构造函数,并将子类的属性作为参数传递给父类构造函数。例如:
```
public class Parent {
protected int parentProperty;
public Parent(int parentProperty) {
this.parentProperty = parentProperty;
}
}
public class Child extends Parent {
private int childProperty;
public Child(int parentProperty, int childProperty) {
super(parentProperty);
this.childProperty = childProperty;
}
public void setParentProperty(int parentProperty) {
super.parentProperty = parentProperty;
}
}
```
在这个例子中,子类Child继承了父类Parent,并在其构造函数中调用了父类的构造函数,将parentProperty传递给了父类。同时,子类Child也有自己的属性childProperty。如果您想将子类的属性值赋给父类的属性,可以使用setParentProperty方法来访问父类的parentProperty属性,并将子类的属性值赋给它。在这个例子中,我们使用了super关键字来访问父类的属性。
java this 子类调父类,父类再调用子类已覆盖的方法及属性(又一次理解)
在Java中,使用关键字`super`可以调用父类的方法或属性,而使用`this`关键字可以引用当前对象的属性或方法。当子类继承了父类并覆盖了父类的方法时,如果想要在父类中调用子类已覆盖的方法,可以使用`super`关键字。
示例代码如下:
```java
class Parent {
void method() {
System.out.println("父类方法");
}
}
class Child extends Parent {
@Override
void method() {
System.out.println("子类方法");
}
void callParentMethod() {
super.method(); // 调用父类方法
}
void callChildMethod() {
this.method(); // 调用子类方法
}
void callOverriddenMethod() {
super.method(); // 调用父类方法
this.method(); // 调用子类方法
}
}
```
在上述示例代码中,子类`Child`覆盖了父类`Parent`的方法`method()`。在子类中,可以使用`super.method()`调用父类的方法,也可以使用`this.method()`调用子类的方法。如果想要在父类中调用子类已覆盖的方法,可以在子类中定义另一个方法,该方法中先使用`super.method()`调用父类的方法,再使用`this.method()`调用子类的方法。
阅读全文
相关推荐
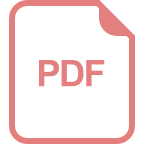
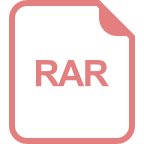
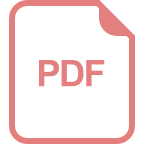
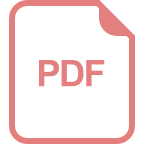
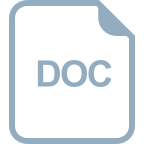
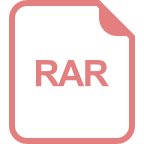
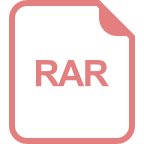





