C++ 对文件锁上解锁
时间: 2023-07-11 12:25:59 浏览: 299
C++ 中可以使用文件锁(File Lock)来实现对文件的锁定和解锁,以防止多个进程同时访问同一文件时出现冲突。C++ 中可以使用 `<fcntl.h>` 头文件中的 `open` 和 `fcntl` 函数来实现文件锁定和解锁。下面是一个示例代码:
```c++
#include <iostream>
#include <fstream>
#include <fcntl.h>
#include <unistd.h>
int lockFile(int fd)
{
struct flock fl;
fl.l_type = F_WRLCK;
fl.l_whence = SEEK_SET;
fl.l_start = 0;
fl.l_len = 0;
return fcntl(fd, F_SETLK, &fl);
}
int unlockFile(int fd)
{
struct flock fl;
fl.l_type = F_UNLCK;
fl.l_whence = SEEK_SET;
fl.l_start = 0;
fl.l_len = 0;
return fcntl(fd, F_SETLK, &fl);
}
int main()
{
std::string filePath = "path/to/file";
// 打开文件
int fd = open(filePath.c_str(), O_RDWR);
if (fd == -1)
{
std::cout << "Failed to open file: " << filePath << std::endl;
return -1;
}
// 锁定文件
if (lockFile(fd) == -1)
{
std::cout << "Failed to lock file: " << filePath << std::endl;
close(fd);
return -1;
}
// 对文件进行读写操作...
std::ofstream file(filePath);
if (file.is_open())
{
file << "Hello, world!" << std::endl;
file.close();
}
// 解锁文件
if (unlockFile(fd) == -1)
{
std::cout << "Failed to unlock file: " << filePath << std::endl;
close(fd);
return -1;
}
// 关闭文件
close(fd);
return 0;
}
```
在上面的示例代码中,`lockFile` 函数用于对文件进行锁定,`unlockFile` 函数用于对文件进行解锁。这两个函数都是通过调用 `fcntl` 函数来实现的。在锁定文件时,我们使用 `F_WRLCK` 参数来设置写锁;在解锁文件时,我们使用 `F_UNLCK` 参数来释放锁。文件锁定成功后,我们可以对文件进行读写操作;在操作完成后,我们需要对文件进行解锁。
需要注意的是,文件锁只能在同一个系统中的不同进程之间起作用,不能在不同系统之间起作用。因此,在使用文件锁时需要确保所有相关的进程都运行在同一台计算机上。
阅读全文
相关推荐
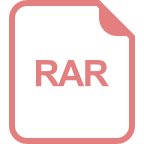















