代码解释 public static boolean ping(String ipAddr, int times, StringBuffer output) { BufferedReader br = null; String cmd = "ping -c " + times + " " + ipAddr; Process process = null; boolean result = false; try { process = Runtime.getRuntime().exec(cmd); if (process == null) { appendLine(output, "ping fail:process is null."); return false; } br = new BufferedReader(new InputStreamReader(process.getInputStream())); while (true) { String line = br.readLine(); if (line == null) { break; } appendLine(output, line); } if (process.waitFor() == 0) { appendLine(output, "exec cmd success:" + cmd); result = true; } else { appendLine(output, "exec cmd fail."); } appendLine(output, "exec finished."); return result; } catch (Exception e) { e.printStackTrace(); return false; } finally { if (process != null) { process.destroy(); } try { if (br != null) br.close(); } catch (IOException unused8) { return result; } } } private static void appendLine(StringBuffer sb, String line) { if (sb != null) { sb.append(line + "\n"); } }
时间: 2023-05-21 18:03:56 浏览: 178
这是一个 Java 方法,用于 ping 指定的 IP 地址,并返回是否成功。其中,参数 ipAddr 是要 ping 的 IP 地址,times 是 ping 的次数,output 是用于输出结果的 StringBuffer 对象。方法中使用 Runtime.getRuntime().exec() 方法执行 ping 命令,并通过 BufferedReader 读取命令执行的结果。如果命令执行成功,返回 true,否则返回 false。方法中还定义了一个私有的 appendLine() 方法,用于将字符串追加到 StringBuffer 对象中。
相关问题
public class IsSubStringDemo { public static boolean isSubString(String str, String subStr) { int strLen = str.length(); int subStrLen = subStr.length(); if (strLen < subStrLen) { return false; } for (int i = 0; i <= strLen - subStrLen; i++) { if (str.substring(i, i + subStrLen).equals(subStr)) { return true; } } return false; } } import java.io.*; public class CountThe { public static void main(String[] args) throws IOException { File file = new File("zuowen.txt"); // 请替换成你的文件路径 BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF-8")); StringBuffer buffer = new StringBuffer(); String line; int count = 0; while ((line = reader.readLine()) != null) { buffer.append(line); } String text = buffer.toString(); for (int i = 0; i < text.length(); i++) { if (text.charAt(i) == '的') { count++; } } System.out.println("文档中共有" + count + "个“的”字。"); } } 怎样更改才能运行
你需要将两个类的代码合并到同一个文件中,像这样:
```
import java.io.*;
public class IsSubStringDemo {
public static boolean isSubString(String str, String subStr) {
int strLen = str.length();
int subStrLen = subStr.length();
if (strLen < subStrLen) {
return false;
}
for (int i = 0; i <= strLen - subStrLen; i++) {
if (str.substring(i, i + subStrLen).equals(subStr)) {
return true;
}
}
return false;
}
public static void main(String[] args) throws IOException {
File file = new File("zuowen.txt"); // 请替换成你的文件路径
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream(file), "UTF-8"));
StringBuffer buffer = new StringBuffer();
String line;
int count = 0;
while ((line = reader.readLine()) != null) {
buffer.append(line);
}
String text = buffer.toString();
for (int i = 0; i < text.length(); i++) {
if (text.charAt(i) == '的') {
count++;
}
}
System.out.println("文档中共有" + count + "个“的”字。");
}
}
```
然后运行这个文件即可。
public static boolean internalIp(String ip) { byte[] addr = textToNumericFormatV4(ip); boolean b = false; if (StringUtils.isNull(addr) || addr.length < 2) { b = true; } else { b = internalIp(addr); } return b || "127.0.0.1".equals(ip); }可能会抛出“NullPointerException”;"addr"在这里可为空。 怎么优化这段代码
可以在方法中添加一个判断语句,检查addr是否为空。如果addr为空,则直接返回true,否则继续执行后面的逻辑。修改后的代码如下:
```
public static boolean internalIp(String ip) {
byte[] addr = textToNumericFormatV4(ip);
if (StringUtils.isNull(addr) || addr.length < 2) {
return true;
}
boolean b = internalIp(addr);
return b || "127.0.0.1".equals(ip);
}
```
这样就可以避免在调用textToNumericFormatV4方法后出现NullPointerException异常,同时也让代码更加简洁易懂。
阅读全文
相关推荐
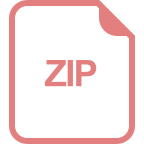
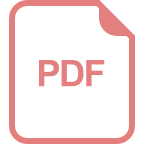
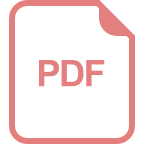
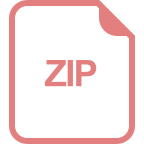
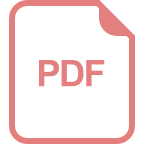
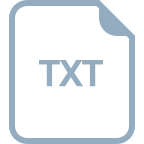
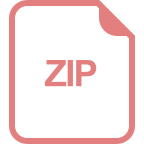
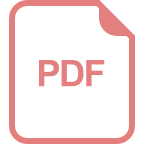
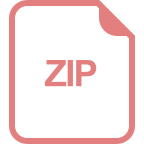
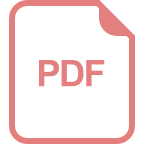
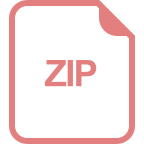
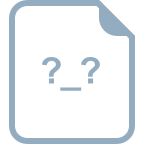
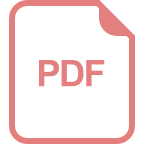
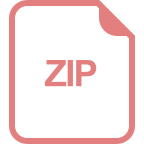
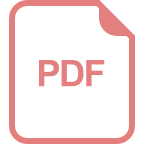

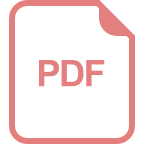