多线程访问volatile关键字修饰的资源有什么问题
时间: 2023-03-19 17:25:23 浏览: 142
多线程访问volatile关键字修饰的资源可能存在可见性问题和原子性问题。因为volatile关键字可以保证被修饰的变量对所有线程的可见性,但是它不能保证对变量的操作具有原子性,也就是说,多个线程同时访问volatile变量时,可能会发生竞争条件的问题。因此,如果需要同时保证可见性和原子性,可以考虑使用synchronized关键字或者使用Java.util.concurrent包提供的原子类来实现。
相关问题
你能举一个,多线程访问volatile关键字修饰资源的例子吗?
### 回答1:
当多个线程访问同一个volatile变量时,如果其中一个线程修改了该变量的值,那么其他线程能够立即看到这个修改。这就是volatile关键字的作用。
以下是一个简单的例子,多个线程并发地访问一个volatile变量:
```
public class VolatileExample {
private volatile int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
VolatileExample example = new VolatileExample();
// 创建10个线程并发执行increment方法
for (int i = 0; i < 10; i++) {
new Thread(() -> {
for (int j = 0; j < 1000; j++) {
example.increment();
}
}).start();
}
// 等待所有线程执行完毕
Thread.sleep(1000);
// 输出最终的count值
System.out.println(example.getCount());
}
}
```
在这个例子中,多个线程并发执行increment方法,每次调用increment方法都会将count值加1。由于count变量被volatile修饰,因此当某个线程修改了count的值时,其他线程能够立即看到这个修改。最终输出的count值应该是10000。
### 回答2:
当一个多线程程序中有一个共享的资源需要被多个线程访问和修改时,我们可以使用volatile关键字来确保线程之间的可见性和同步操作。下面是一个使用volatile关键字的例子:
假设有一个计数器变量count,多个线程同时对其进行自增操作,代码如下:
```java
public class VolatileExample {
private volatile int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
```
在这个例子中,count变量被volatile关键字修饰,这表示该变量在多线程中的访问是可见的。当一个线程对count进行自增操作时,其他线程能够立即看到更新后的值。如果没有使用volatile关键字修饰count变量,可能会出现线程之间访问到过期的值的情况,导致计数器的结果不准确。
例如,有两个线程Thread1和Thread2同时访问VolatileExample对象的increment方法:
```java
public static void main(String[] args) {
VolatileExample example = new VolatileExample();
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
example.increment();
}
});
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Count: " + example.getCount());
}
```
运行以上代码,输出的Count结果应该为2000。这是因为VolatileExample对象的count变量被volatile修饰,确保了在线程之间的可见性和同步操作,从而保证了计数器的准确性。
### 回答3:
多线程访问volatile关键字修饰资源的一个例子可以是计数器的实现。
假设有一个计数器类Counter,其中的count变量使用volatile关键字修饰,表示多个线程都能够正确读取和写入该变量。以下是一个简单的示例代码:
```
public class Counter {
private volatile int count;
public Counter() {
this.count = 0;
}
public int getCount() {
return count;
}
public void increment() {
this.count++;
}
}
public class Main {
public static void main(String[] args) {
Counter counter = new Counter();
// 创建多个线程同时对计数器进行操作
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
counter.increment();
}
});
t1.start();
t2.start();
// 等待两个线程执行完毕
try {
t1.join();
t2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
// 输出计数器的值
System.out.println("Count: " + counter.getCount());
}
}
```
在上述代码中,创建了两个线程t1和t2,分别对计数器进行1000次递增操作。由于count变量使用volatile关键字修饰,所以两个线程能够正确地读取和写入该变量的最新值,确保了计数器的正确性。最后,输出计数器的值为2000,说明两个线程分别进行了1000次递增操作。
这个例子展示了多线程访问volatile关键字修饰资源的使用方法和效果,可以保证多个线程之间对共享资源的可见性和一致性。
使用volatile 关键字修饰静态变量 实例
可以使用`volatile`关键字修饰静态变量和实例变量。下面是示例代码:
```java
public class MyClass {
public static volatile int staticVar; // 修饰静态变量
public volatile int instanceVar; // 修饰实例变量
}
```
通过使用`volatile`关键字修饰静态变量和实例变量,可以保证多个线程之间对变量访问的可见性。但需要注意的是,`volatile`并不能保证原子性,如果需要原子性,需要使用`synchronized`或`java.util.concurrent.atomic`包下的原子类。
阅读全文
相关推荐
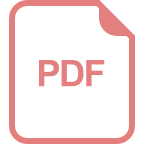
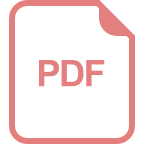
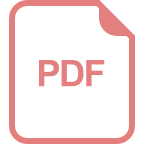
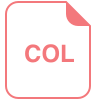
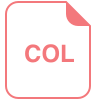
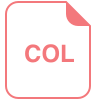
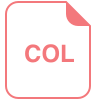








