C# 根据实体类名获取所有字段对应的数据库字段名
时间: 2024-05-05 20:22:42 浏览: 15
可以使用反射获取实体类中所有字段的属性,然后通过自定义属性或者约定俗成的规则,获取每个字段对应的数据库字段名。
以下是一个示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
public static class DatabaseUtility
{
public static Dictionary<string, string> GetDatabaseFields(string entityClassName, string prefix = "")
{
var entityAssembly = Assembly.GetExecutingAssembly(); // 获取实体类所在的程序集
var entityClass = entityAssembly.GetTypes().FirstOrDefault(t => t.Name == entityClassName); // 获取实体类的 Type
if (entityClass == null)
{
throw new ArgumentException($"Entity class {entityClassName} not found in assembly.");
}
var databaseFields = new Dictionary<string, string>();
foreach (var field in entityClass.GetFields(BindingFlags.Public | BindingFlags.Instance))
{
var fieldName = prefix + field.Name; // 默认情况下,数据库字段名与实体类字段名相同,可以通过参数 prefix 添加前缀
var databaseFieldAttribute = field.GetCustomAttribute<DatabaseFieldAttribute>();
if (databaseFieldAttribute != null)
{
fieldName = databaseFieldAttribute.FieldName;
}
databaseFields.Add(field.Name, fieldName);
}
return databaseFields;
}
}
// 自定义属性,用于标记实体类中的字段对应的数据库字段名
[AttributeUsage(AttributeTargets.Field)]
public class DatabaseFieldAttribute : Attribute
{
public string FieldName { get; }
public DatabaseFieldAttribute(string fieldName)
{
FieldName = fieldName;
}
}
```
使用示例:
```csharp
public class User
{
[DatabaseField("id")]
public int Id;
[DatabaseField("username")]
public string Username;
[DatabaseField("password")]
public string Password;
}
var databaseFields = DatabaseUtility.GetDatabaseFields("User", "u_");
foreach (var pair in databaseFields)
{
Console.WriteLine($"Entity field {pair.Key} maps to database field {pair.Value}.");
}
// 输出:
// Entity field Id maps to database field u_id.
// Entity field Username maps to database field u_username.
// Entity field Password maps to database field u_password.
```
注意:上述代码中使用了自定义属性 `DatabaseFieldAttribute`,可以根据实际情况进行修改。如果没有自定义属性,可以约定俗成地将实体类字段名转为小写,然后添加下划线前缀作为数据库字段名。
相关推荐
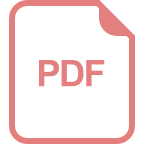
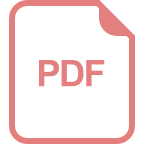










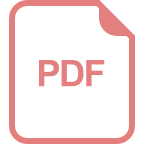