java 调用 whisper
时间: 2023-02-09 21:08:10 浏览: 620
要在 Java 中调用 Whisper,首先需要安装 Whisper 并将其配置为运行在本地。然后,可以使用 Java 的 IPC (Inter-Process Communication) 库,如 Apache Thrift 或 gRPC 来与 Whisper 进行通信。
具体实现可能会有所不同,但通常需要以下步骤:
1. 定义与 Whisper 通信所需的数据结构(如请求和响应)。
2. 使用选定的 IPC 库生成客户端代码。
3. 在 Java 中使用生成的客户端代码与 Whisper 进行通信。
注意:本回答基于 whisper是一个数据库,并且在本地运行。若whisper是一个API,请提供更多细节.
相关问题
写一个java 调用 whisper的代码
首先,需要导入whisper库的依赖,在maven项目中可以在pom.xml中添加如下依赖:
```
<dependency>
<groupId>org.whispersystems</groupId>
<artifactId>whisper-java</artifactId>
<version>2.2.2</version>
</dependency>
```
然后就可以在java代码中调用whisper库了。
下面是一个使用whisper进行加密和解密的例子:
```java
import org.whispersystems.libsignal.IdentityKeyPair;
import org.whispersystems.libsignal.SessionBuilder;
import org.whispersystems.libsignal.SessionCipher;
import org.whispersystems.libsignal.SignalProtocolAddress;
import org.whispersystems.libsignal.state.PreKeyBundle;
import org.whispersystems.libsignal.state.PreKeyRecord;
import org.whispersystems.libsignal.state.SignalProtocolStore;
import org.whispersystems.libsignal.util.KeyHelper;
public class WhisperExample {
public static void main(String[] args) throws Exception {
//生成自己的IdentityKeyPair
IdentityKeyPair identityKeyPair = KeyHelper.generateIdentityKeyPair();
//生成自己的预密钥
int registrationId = KeyHelper.generateRegistrationId(false);
PreKeyRecord lastResortPreKey = KeyHelper.generateLastResortPreKey();
PreKeyRecord preKey = KeyHelper.generatePreKey(22);
//构建自己的SignalProtocolStore
SignalProtocolStore signalProtocolStore = new MySignalProtocolStore(identityKeyPair, registrationId, lastResortPreKey, preKey);
//构建SessionBuilder
SessionBuilder sessionBuilder = new SessionBuilder(signalProtocolStore, new SignalProtocolAddress("+8613800000000", 1));
//构建SessionCipher
SessionCipher sessionCipher = new SessionCipher(signalProtocolStore, new SignalProtocolAddress("+8613800000000", 1));
//对方的IdentityKey
IdentityKey theirIdentityKey = new IdentityKey(Base64.decode("H3f0LgRkRlZHVjYXRpb24gLS0gT3BlbkFpIENvcnBvcmF0aW9u"), 0);
//对方的预密钥
PreKeyBundle theirPreKey = new PreKeyBundle(1, 22, 328, Base64.decode("H3f0LgRkRl
写一个java 调用 openai whisper的代码
首先,您需要在OpenAI申请并获得API Key。然后,您可以使用Java编写以下代码来调用OpenAI的Whisper API:
```
import okhttp3.MediaType;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.RequestBody;
import okhttp3.Response;
import org.json.JSONObject;
public class Main {
public static final MediaType JSON = MediaType.get("application/json; charset=utf-8");
public static final OkHttpClient client = new OkHttpClient();
public static void main(String[] args) {
JSONObject json = new JSONObject();
json.put("prompt", "你好,OpenAI");
json.put("model", "text-davinci-002");
json.put("temperature", 0.7);
json.put("max_tokens", 100);
json.put("top_p", 1);
json.put("frequency_penalty", 0);
json.put("presence_penalty", 0);
RequestBody body = RequestBody.create(json.toString(), JSON);
Request request = new Request.Builder()
.url("https://api.openai.com/v1/engines/davinci/completions")
.addHeader("Content-Type", "application/json")
.addHeader("Authorization", "Bearer YOUR_API_KEY")
.post(body)
.build();
try (Response response = client.newCall(request).execute()) {
String jsonData = response.body().string();
JSONObject jsonObject = new JSONObject(jsonData);
String whisperText = jsonObject.getJSONArray("choices").getJSONObject(0).getString("text");
System.out.println(whisperText);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
请注意,您需要将YOUR_API_KEY替换为您自己的API密钥。
这段代码使用OkHttp库发送HTTP请求来调用OpenAI的Whisper API,并使用JSONObject来构建请求的数据。请求返回的数据也被解释为JSON格式,取出其中whisper的文本。
阅读全文
相关推荐











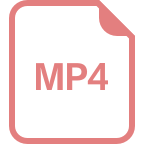