如何使用Visual Studio编写一个程序来接收用户输入的语文、数学、英语三科成绩,然后计算总分和平均分并将其打印出来?
时间: 2024-10-25 13:07:53 浏览: 15
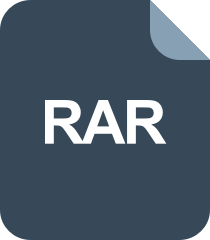
visual studio 2010编写语文数学英语三科成绩
在Visual Studio中创建一个简单的控制台应用程序来完成这个任务,可以按照以下步骤进行:
1. **打开Visual Studio**:
打开Visual Studio IDE,选择新建项目,然后选择“控制台应用”作为模板。
2. **添加所需命名空间**:
在`Program.cs`文件开头,添加`using System;`以便使用内置的Console类进行交互。
3. **设计类结构**:
定义一个名为`Score`的简单类,包含属性如`Chinese`, `Math`, 和 `English`,表示各科的成绩,以及`TotalScore`和`AverageScore`两个辅助属性。
```csharp
public class Score
{
public int Chinese { get; set; }
public int Math { get; set; }
public int English { get; set; }
private int TotalScore { get => Chinese + Math + English; }
private double AverageScore { get => (double)TotalScore / 3; }
}
```
4. **主函数**:
在`Main`函数中,通过`Console.ReadLine()`获取用户的输入,转换为整数,并实例化`Score`对象。
```csharp
static void Main(string[] args)
{
Console.WriteLine("请输入语文成绩:");
int chinese = Int32.Parse(Console.ReadLine());
Console.WriteLine("请输入数学成绩:");
int math = Int32.Parse(Console.ReadLine());
Console.WriteLine("请输入英语成绩:");
int english = Int32.Parse(Console.ReadLine());
Score userScore = new Score
{
Chinese = chinese,
Math = math,
English = english
};
// 计算并打印结果
Console.WriteLine($"总分:{userScore.TotalScore}");
Console.WriteLine($"平均分:{userScore.AverageScore}");
}
```
5. **运行程序**:
点击运行按钮或者按F5键,程序会提示用户输入三个科目的成绩,然后显示总分和平均分。
阅读全文
相关推荐
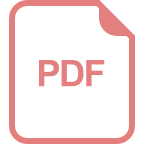
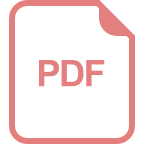
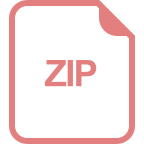
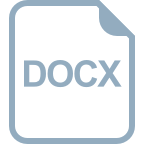
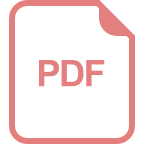
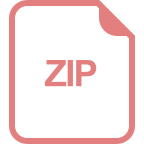
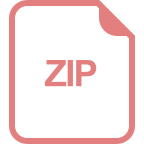
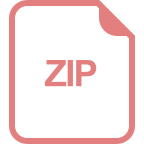
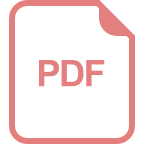
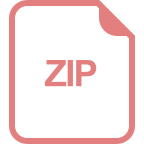
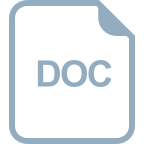