用django和matplotlib ,在web页面查找不同的可视化图片,写出详细代码
时间: 2023-06-09 16:06:33 浏览: 56
好的,以下是用django和matplotlib,在web页面查找不同的可视化图片的详细代码:
首先安装必要的依赖,包括django和matplotlib:
```
pip install django
pip install matplotlib
```
然后在django项目中创建一个名为`graph`的应用程序:
```
python manage.py startapp graph
```
在`graph`应用程序中创建一个`views.py`文件,编写如下代码:
```python
from django.http import HttpResponse
from django.shortcuts import render
import matplotlib.pyplot as plt
import io
import urllib, base64
def index(request):
# 生成一个简单的折线图
plt.plot([1, 2, 3, 4, 5], [1, 2, 3, 4, 5])
plt.ylabel('y')
plt.xlabel('x')
plt.title('Graph')
# 将图片保存为二进制字符串
buffer = io.BytesIO()
plt.savefig(buffer, format='png')
buffer.seek(0)
image_png = buffer.getvalue()
buffer.close()
# 将二进制字符串转换为base64字符串
graphic = base64.b64encode(image_png).decode('utf-8')
# 在web页面上显示图形
context = {'graphic': graphic}
return render(request, "graph/index.html", context)
```
这个视图函数生成一个简单的折线图,并将其转换为base64字符串以便在web页面上显示。然后在`graph`应用程序中创建一个名为`index.html`的模板文件,编写如下代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>Graph</title>
</head>
<body>
{% if graphic %}
<img src="data:image/png;base64,{{ graphic }}">
{% endif %}
</body>
</html>
```
这个模板文件包含一个用于显示图形的图像元素,并使用base64字符串作为图像源。
最后,在django项目的URLconf中添加一个URL模式:
```python
from django.urls import path
from graph.views import index
urlpatterns = [
path('', index, name='index'),
]
```
现在运行django开发服务器,打开浏览器并访问`http://localhost:8000/`,即可看到一个简单的折线图。
请注意,这只是一个演示,你可以根据需要更改代码以生成不同的图形并在web页面上进行显示。
相关推荐
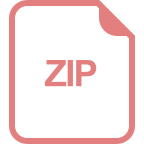
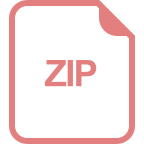
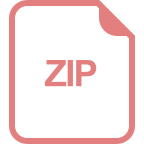
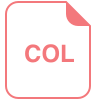
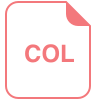
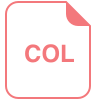
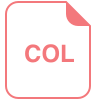
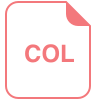









