Ehcache如何定时刷新数据
时间: 2023-08-10 15:07:25 浏览: 255
Ehcache 提供了两种定时刷新数据的方式:定时刷新和定时过期。
1. 定时刷新
定时刷新是指在缓存到期之前,定期地刷新缓存中的数据。这样可以保证缓存数据始终是最新的。Ehcache提供了一个`CacheExtension`接口,可以用来实现定时刷新。具体实现步骤如下:
- 创建一个类,实现`CacheExtension`接口,并重写`init`和`dispose`方法。
- 在`init`方法中启动一个定时任务,定期刷新缓存中的数据。
- 在`dispose`方法中停止定时任务。
下面是一个简单的定时刷新实现示例:
```java
public class MyCacheExtension implements CacheExtension<Object, Object> {
private ScheduledExecutorService scheduler = Executors.newSingleThreadScheduledExecutor();
private final Cache<Object, Object> cache;
public MyCacheExtension(Cache<Object, Object> cache) {
this.cache = cache;
}
@Override
public void init() throws CacheException {
scheduler.scheduleAtFixedRate(() -> {
// 定时刷新缓存中的数据
// ...
}, 0, 1, TimeUnit.MINUTES); // 每1分钟刷新一次
}
@Override
public void dispose() throws CacheException {
scheduler.shutdown();
}
@Override
public Object get(Object key) throws CacheException {
return cache.get(key);
}
@Override
public void put(Object key, Object value) throws CacheException {
cache.put(key, value);
}
@Override
public boolean remove(Object key) throws CacheException {
return cache.remove(key);
}
@Override
public void clear() throws CacheException {
cache.clear();
}
}
```
2. 定时过期
定时过期是指在固定的时间点,缓存中的数据自动过期。Ehcache提供了`timeToLiveSeconds`和`timeToIdleSeconds`两个属性来配置缓存数据的过期时间。`timeToLiveSeconds`表示缓存数据的存活时间,即从缓存放入时间开始计算,缓存数据在指定时间后自动过期;`timeToIdleSeconds`表示缓存数据的空闲时间,即从缓存最后一次访问时间开始计算,缓存数据在指定时间后自动过期。
下面是一个定时过期的示例:
```java
CacheConfiguration configuration = new CacheConfiguration();
configuration.setName("myCache");
configuration.setMaxEntriesLocalHeap(1000);
configuration.setTimeToLiveSeconds(60); // 设置缓存数据的存活时间为60秒
configuration.setTimeToIdleSeconds(30); // 设置缓存数据的空闲时间为30秒
CacheManager cacheManager = CacheManagerBuilder.newCacheManagerBuilder()
.withCache("myCache", configuration)
.build(true);
Cache<Object, Object> cache = cacheManager.getCache("myCache", Object.class, Object.class);
cache.put("key1", "value1");
Thread.sleep(40000); // 等待40秒
Object value = cache.get("key1"); // 此时返回null,缓存数据已自动过期
```
阅读全文
相关推荐
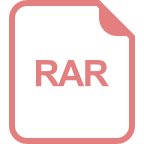
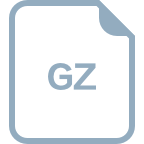
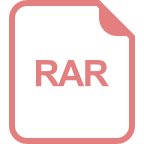
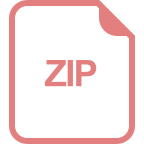
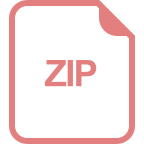
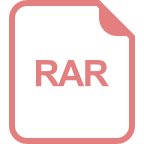
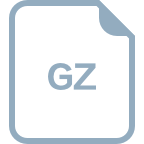
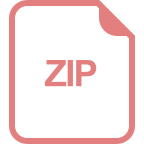
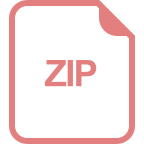
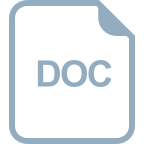
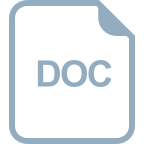
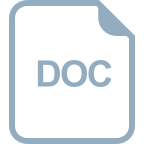
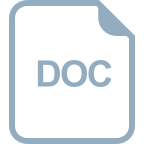
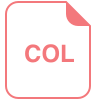
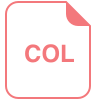
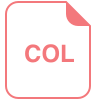
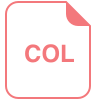