头哥java继承和多态之综合练习
时间: 2023-11-12 17:05:11 浏览: 149
好的,下面给你一个综合练习,希望能够帮助你熟悉Java继承和多态的使用。
题目描述:
设计一个简单的员工管理系统,要求实现员工信息录入、查看、修改和删除等功能。该系统包含以下员工类型:
1.普通员工:包含员工编号、姓名、性别、年龄、职位、基本工资等属性,以及计算薪水的方法(基本工资 + 奖金)。
2.经理:包含员工编号、姓名、性别、年龄、职位、基本工资、奖金、部门等属性,以及计算薪水的方法(基本工资 + 奖金 + 年度绩效)。
3.销售员:包含员工编号、姓名、性别、年龄、职位、基本工资、销售额、提成比例等属性,以及计算薪水的方法(基本工资 + 销售额 * 提成比例)。
要求:
1.使用继承和多态的概念实现员工类的设计。
2.使用ArrayList集合来存储员工信息。
3.实现员工信息的增删改查功能。
4.实现员工薪水的计算和显示功能。
5.使用菜单方式来实现系统的交互。
代码实现:
首先,我们需要先定义一个父类Employee,包含员工编号、姓名、性别、年龄、职位、基本工资等属性,以及计算薪水的方法(基本工资 + 奖金)。
```java
public class Employee {
private String id; // 员工编号
private String name; // 姓名
private String sex; // 性别
private int age; // 年龄
private String position; // 职位
private double salary; // 基本工资
public Employee(String id, String name, String sex, int age, String position, double salary) {
this.id = id;
this.name = name;
this.sex = sex;
this.age = age;
this.position = position;
this.salary = salary;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPosition() {
return position;
}
public void setPosition(String position) {
this.position = position;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public double calcSalary() {
return salary;
}
}
```
接下来,我们分别定义三个子类,分别为Manager、Salesman和NormalEmployee,分别添加各自特有的属性和计算薪水的方法。
```java
public class Manager extends Employee {
private double bonus; // 奖金
private double performance; // 年度绩效
private String department; // 部门
public Manager(String id, String name, String sex, int age, String position, double salary, double bonus,
double performance, String department) {
super(id, name, sex, age, position, salary);
this.bonus = bonus;
this.performance = performance;
this.department = department;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
public double getPerformance() {
return performance;
}
public void setPerformance(double performance) {
this.performance = performance;
}
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
@Override
public double calcSalary() {
return super.calcSalary() + bonus + performance;
}
}
```
```java
public class Salesman extends Employee {
private double sales; // 销售额
private double commission; // 提成比例
public Salesman(String id, String name, String sex, int age, String position, double salary, double sales,
double commission) {
super(id, name, sex, age, position, salary);
this.sales = sales;
this.commission = commission;
}
public double getSales() {
return sales;
}
public void setSales(double sales) {
this.sales = sales;
}
public double getCommission() {
return commission;
}
public void setCommission(double commission) {
this.commission = commission;
}
@Override
public double calcSalary() {
return super.calcSalary() + sales * commission;
}
}
```
```java
public class NormalEmployee extends Employee {
public NormalEmployee(String id, String name, String sex, int age, String position, double salary) {
super(id, name, sex, age, position, salary);
}
}
```
接下来,我们需要实现员工信息的增删改查功能,以及员工薪水的计算和显示功能,具体实现如下:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class EmployeeSystem {
private ArrayList<Employee> employees = new ArrayList<>(); // 员工列表
// 添加员工
public void addEmployee(Employee employee) {
employees.add(employee);
}
// 删除员工
public void deleteEmployee(String id) {
for (int i = 0; i < employees.size(); i++) {
Employee employee = employees.get(i);
if (employee.getId().equals(id)) {
employees.remove(i);
break;
}
}
}
// 修改员工
public void updateEmployee(String id, Employee employee) {
for (int i = 0; i < employees.size(); i++) {
Employee e = employees.get(i);
if (e.getId().equals(id)) {
e.setName(employee.getName());
e.setSex(employee.getSex());
e.setAge(employee.getAge());
e.setPosition(employee.getPosition());
e.setSalary(employee.getSalary());
if (employee instanceof Manager) {
Manager manager = (Manager) employee;
((Manager) e).setBonus(manager.getBonus());
((Manager) e).setPerformance(manager.getPerformance());
((Manager) e).setDepartment(manager.getDepartment());
} else if (employee instanceof Salesman) {
Salesman salesman = (Salesman) employee;
((Salesman) e).setSales(salesman.getSales());
((Salesman) e).setCommission(salesman.getCommission());
}
break;
}
}
}
// 查询员工
public Employee queryEmployee(String id) {
for (int i = 0; i < employees.size(); i++) {
Employee employee = employees.get(i);
if (employee.getId().equals(id)) {
return employee;
}
}
return null;
}
// 计算所有员工的薪水
public double calcAllSalary() {
double totalSalary = 0;
for (int i = 0; i < employees.size(); i++) {
Employee employee = employees.get(i);
totalSalary += employee.calcSalary();
}
return totalSalary;
}
// 显示所有员工的信息
public void showAllEmployees() {
for (int i = 0; i < employees.size(); i++) {
Employee employee = employees.get(i);
System.out.println("员工编号:" + employee.getId());
System.out.println("姓名:" + employee.getName());
System.out.println("性别:" + employee.getSex());
System.out.println("年龄:" + employee.getAge());
System.out.println("职位:" + employee.getPosition());
System.out.println("工资:" + employee.calcSalary());
System.out.println("--------------------------");
}
}
// 显示菜单
public void showMenu() {
System.out.println("==========员工管理系统==========");
System.out.println("1.添加员工");
System.out.println("2.删除员工");
System.out.println("3.修改员工");
System.out.println("4.查询员工");
System.out.println("5.显示所有员工");
System.out.println("6.计算所有员工的薪水");
System.out.println("0.退出系统");
System.out.println("请选择操作:");
}
public static void main(String[] args) {
EmployeeSystem es = new EmployeeSystem();
Scanner scanner = new Scanner(System.in);
while (true) {
es.showMenu();
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入员工类型(1.普通员工 2.经理 3.销售员):");
int type = scanner.nextInt();
System.out.println("请输入员工编号:");
String id = scanner.next();
System.out.println("请输入员工姓名:");
String name = scanner.next();
System.out.println("请输入员工性别:");
String sex = scanner.next();
System.out.println("请输入员工年龄:");
int age = scanner.nextInt();
System.out.println("请输入员工职位:");
String position = scanner.next();
System.out.println("请输入员工基本工资:");
double salary = scanner.nextDouble();
Employee employee = null;
if (type == 1) {
employee = new NormalEmployee(id, name, sex, age, position, salary);
} else if (type == 2) {
System.out.println("请输入员工奖金:");
double bonus = scanner.nextDouble();
System.out.println("请输入员工年度绩效:");
double performance = scanner.nextDouble();
System.out.println("请输入员工部门:");
String department = scanner.next();
employee = new Manager(id, name, sex, age, position, salary, bonus, performance, department);
} else if (type == 3) {
System.out.println("请输入员工销售额:");
double sales = scanner.nextDouble();
System.out.println("请输入员工提成比例:");
double commission = scanner.nextDouble();
employee = new Salesman(id, name, sex, age, position, salary, sales, commission);
}
es.addEmployee(employee);
System.out.println("添加成功!");
break;
case 2:
System.out.println("请输入员工编号:");
id = scanner.next();
es.deleteEmployee(id);
System.out.println("删除成功!");
break;
case 3:
System.out.println("请输入员工编号:");
id = scanner.next();
employee = es.queryEmployee(id);
if (employee == null) {
System.out.println("员工不存在!");
} else {
System.out.println("请输入员工姓名:");
name = scanner.next();
System.out.println("请输入员工性别:");
sex = scanner.next();
System.out.println("请输入员工年龄:");
age = scanner.nextInt();
System.out.println("请输入员工职位:");
position = scanner.next();
System.out.println("请输入员工基本工资:");
salary = scanner.nextDouble();
if (employee instanceof Manager) {
System.out.println("请输入员工奖金:");
double bonus = scanner.nextDouble();
System.out.println("请输入员工年度绩效:");
double performance = scanner.nextDouble();
System.out.println("请输入员工部门:");
String department = scanner.next();
Manager manager = new Manager(id, name, sex, age, position, salary, bonus, performance,
department);
es.updateEmployee(id, manager);
} else if (employee instanceof Salesman) {
System.out.println("请输入员工销售额:");
double sales = scanner.nextDouble();
System.out.println("请输入员工提成比例:");
double commission = scanner.nextDouble();
Salesman salesman = new Salesman(id, name, sex, age, position, salary, sales, commission);
es.updateEmployee(id, salesman);
} else {
NormalEmployee normalEmployee = new NormalEmployee(id, name, sex, age, position, salary);
es.updateEmployee(id, normalEmployee);
}
System.out.println("修改成功!");
}
break;
case 4:
System.out.println("请输入员工编号:");
id = scanner.next();
employee = es.queryEmployee(id);
if (employee == null) {
System.out.println("员工不存在!");
} else {
System.out.println("员工编号:" + employee.getId());
System.out.println("姓名:" + employee.getName());
System.out.println("性别:" + employee.getSex());
System.out.println("年龄:" + employee.getAge());
System.out.println("职位:" + employee.getPosition());
System.out.println("工资:" + employee.calcSalary());
}
break;
case 5:
es.showAllEmployees();
break;
case 6:
double totalSalary = es.calcAllSalary();
System.out.println("所有员工的薪水总和为:" + totalSalary);
break;
case 0:
System.exit(0);
default:
System.out.println("输入有误,请重新输入!");
}
}
}
}
```
这样,一个简单的员工管理系统就完成了。你可以尝试运行这个程序,体验一下它的功能。
阅读全文
相关推荐
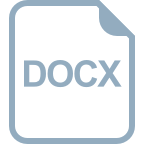















